GitHub - null8626/decancer: A library that removes common unicode confusables/homoglyphs from strings.A library that removes common unicode confusables/homoglyphs from strings. - null8626/decancer
Visit Site
GitHub - null8626/decancer: A library that removes common unicode confusables/homoglyphs from strings.
decancer

A library that removes common unicode confusables/homoglyphs from strings.
- Its core is written in Rust and utilizes a form of Binary Search to ensure speed!
- By default, it's capable of filtering 221,529 (19.88%) different unicode codepoints like:
- All whitespace characters
- All diacritics, this also eliminates all forms of Zalgo text
- Most leetspeak characters
- Most homoglyphs
- Several emojis
- Unlike other packages, this package is unicode bidi-aware where it also interprets right-to-left characters in the same way as it were to be rendered by an application!
- Its behavior is also highly customizable to your liking!
- And it's available in the following languages:
Installation
In your Cargo.toml
:
decancer = "3.2.8"
In your shell:
npm install decancer
In your code (CommonJS):
const decancer = require('decancer')
In your code (ESM):
import decancer from 'decancer'
In your code:
<script type="module">
import init from 'https://cdn.jsdelivr.net/gh/null8626/[email protected]/bindings/wasm/bin/decancer.min.js'
const decancer = await init()
</script>
As a JAR file
You can download the latest JAR file here.
As a dependency
In your build.gradle
:
repositories {
mavenCentral()
maven { url 'https://jitpack.io' }
}
dependencies {
implementation 'io.github.null8626:decancer:3.2.8'
}
In your pom.xml
:
<repositories>
<repository>
<id>central</id>
<url>https://repo.maven.apache.org/maven2</url>
</repository>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>io.github.null8626</groupId>
<artifactId>decancer</artifactId>
<version>3.2.8</version>
</dependency>
</dependencies>
Building from source
Windows:
git clone https://github.com/null8626/decancer.git --depth 1
cd .\decancer\bindings\java
powershell -NoLogo -NoProfile -NonInteractive -Command "Expand-Archive -Path .\bin\bindings.zip -DestinationPath .\bin -Force"
gradle build -x test
macOS/Linux:
git clone https://github.com/null8626/decancer.git --depth 1
cd ./decancer/bindings/java
unzip ./bin/bindings.zip -d ./bin
chmod +x ./gradlew
./gradlew build -x test
Tip: You can shrink the size of the resulting JAR file by removing binaries in the bin
directory for the platforms you don't want to support.
Download
- Header file
- Download for ARM64 macOS (11.0+, Big Sur+)
- Download for ARM64 iOS
- Download for Apple iOS Simulator on ARM6
- Download for ARM64 Android
- Download for ARM64 Windows MSVC
- Download for ARM64 Linux (kernel 4.1, glibc 2.17+)
- Download for ARM64 Linux with MUSL
- Download for ARMv6 Linux (kernel 3.2, glibc 2.17)
- Download for ARMv5TE Linux (kernel 4.4, glibc 2.23)
- Download for ARMv7-A Android
- Download for ARMv7-A Linux (kernel 4.15, glibc 2.27)
- Download for ARMv7-A Linux, hardfloat (kernel 3.2, glibc 2.17)
- Download for 32-bit Linux w/o SSE (kernel 3.2, glibc 2.17)
- Download for 32-bit MSVC (Windows 7+)
- Download for 32-bit FreeBSD
- Download for 32-bit Linux (kernel 3.2+, glibc 2.17+)
- Download for PPC64LE Linux (kernel 3.10, glibc 2.17)
- Download for RISC-V Linux (kernel 4.20, glibc 2.29)
- Download for S390x Linux (kernel 3.2, glibc 2.17)
- Download for SPARC Solaris 11, illumos
- Download for Thumb2-mode ARMv7-A Linux with NEON (kernel 4.4, glibc 2.23)
- Download for 64-bit macOS (10.12+, Sierra+)
- Download for 64-bit iOS
- Download for 64-bit MSVC (Windows 7+)
- Download for 64-bit FreeBSD
- Download for 64-bit illumos
- Download for 64-bit Linux (kernel 3.2+, glibc 2.17+)
- Download for 64-bit Linux with MUSL
Building from source
Building from source requires Rust v1.65 or later.
git clone https://github.com/null8626/decancer.git --depth 1
cd decancer/bindings/native
cargo build --release
And the binary files should be generated in the target/release
directory.
Examples
For more information, please read the documentation.
let mut cured = decancer::cure!(r"vEⓡ𝔂 𝔽𝕌Ňℕy ţ乇𝕏𝓣 wWiIiIIttHh l133t5p3/-\|<").unwrap();
assert_eq!(cured, "very funny text with leetspeak");
// WARNING: it's NOT recommended to coerce this output to a Rust string
// and process it manually from there, as decancer has its own
// custom comparison measures, including leetspeak matching!
assert_ne!(cured.as_str(), "very funny text with leetspeak");
assert!(cured.contains("funny"));
cured.censor("funny", '*');
assert_eq!(cured, "very ***** text with leetspeak");
cured.censor_multiple(["very", "text"], '-');
assert_eq!(cured, "---- ***** ---- with leetspeak");
const assert = require('assert')
const cured = decancer('vEⓡ𝔂 𝔽𝕌Ňℕy ţ乇𝕏𝓣 wWiIiIIttHh l133t5p3/-\\|<')
assert(cured.equals('very funny text with leetspeak'))
// WARNING: it's NOT recommended to coerce this output to a JavaScript string
// and process it manually from there, as decancer has its own
// custom comparison measures, including leetspeak matching!
assert(cured.toString() !== 'very funny text with leetspeak')
console.log(cured.toString())
// => very funny text wwiiiiitthh l133t5p3/-\|<
assert(cured.contains('funny'))
cured.censor('funny', '*')
console.log(cured.toString())
// => very ***** text wwiiiiitthh l133t5p3/-\|<
cured.censorMultiple(['very', 'text'], '-')
console.log(cured.toString())
// => ---- ***** ---- wwiiiiitthh l133t5p3/-\|<
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Decancerer!!! (tm)</title>
<style>
textarea {
font-size: 30px;
}
#cure {
font-size: 20px;
padding: 5px 30px;
}
</style>
</head>
<body>
<h3>Input cancerous text here:</h3>
<textarea rows="10" cols="30"></textarea>
<br />
<button id="cure" onclick="cure()">cure!</button>
<script type="module">
import init from 'https://cdn.jsdelivr.net/gh/null8626/[email protected]/bindings/wasm/bin/decancer.min.js'
const decancer = await init()
window.cure = function () {
const textarea = document.querySelector('textarea')
if (!textarea.value.length) {
return alert("There's no text!!!")
}
textarea.value = decancer(textarea.value).toString()
}
</script>
</body>
</html>
For more information, please read the documentation.
import io.github.null8626.decancer.CuredString;
public class Program {
public static void main(String[] args) {
CuredString cured = new CuredString("vEⓡ𝔂 𝔽𝕌Ňℕy ţ乇𝕏𝓣 wWiIiIIttHh l133t5p3/-\\|<");
assert cured.equals("very funny text with leetspeak");
// WARNING: it's NOT recommended to coerce this output to a Java String
// and process it manually from there, as decancer has its own
// custom comparison measures, including leetspeak matching!
assert !cured.toString().equals("very funny text with leetspeak");
System.out.println(cured.toString());
// => very funny text wwiiiiitthh l133t5p3/-\|<
assert cured.contains("funny");
cured.censor("funny", '*');
System.out.println(cured.toString());
// => very ***** text wwiiiiitthh l133t5p3/-\|<
String[] keywords = { "very", "text" };
cured.censorMultiple(keywords, '-');
System.out.println(cured.toString());
// => ---- ***** ---- wwiiiiitthh l133t5p3/-\|<
cured.destroy();
}
}
For more information, please read the documentation.
UTF-8 example:
#include <decancer.h>
#include <string.h>
#include <stdlib.h>
#include <stdio.h>
#define decancer_assert(expr, notes) \
if (!(expr)) { \
fprintf(stderr, "assertion failure at " notes "\n"); \
ret = 1; \
goto END; \
}
int main(void) {
int ret = 0;
// UTF-8 bytes for "vEⓡ𝔂 𝔽𝕌Ňℕy ţ乇𝕏𝓣"
uint8_t input[] = {0x76, 0xef, 0xbc, 0xa5, 0xe2, 0x93, 0xa1, 0xf0, 0x9d, 0x94, 0x82, 0x20, 0xf0, 0x9d,
0x94, 0xbd, 0xf0, 0x9d, 0x95, 0x8c, 0xc5, 0x87, 0xe2, 0x84, 0x95, 0xef, 0xbd, 0x99,
0x20, 0xc5, 0xa3, 0xe4, 0xb9, 0x87, 0xf0, 0x9d, 0x95, 0x8f, 0xf0, 0x9d, 0x93, 0xa3};
decancer_error_t error;
decancer_cured_t cured = decancer_cure(input, sizeof(input), DECANCER_OPTION_DEFAULT, &error);
if (cured == NULL) {
fprintf(stderr, "curing error: %.*s\n", (int)error.message_length, error.message);
return 1;
}
decancer_assert(decancer_contains(cured, "funny", 5), "decancer_contains");
END:
decancer_cured_free(cured);
return ret;
}
UTF-16 example:
#include <decancer.h>
#include <string.h>
#include <stdlib.h>
#include <stdio.h>
#define decancer_assert(expr, notes) \
if (!(expr)) { \
fprintf(stderr, "assertion failure at " notes "\n"); \
ret = 1; \
goto END; \
}
int main(void) {
int ret = 0;
// UTF-16 bytes for "vEⓡ𝔂 𝔽𝕌Ňℕy ţ乇𝕏𝓣"
uint16_t input[] = {
0x0076, 0xff25, 0x24e1,
0xd835, 0xdd02, 0x0020,
0xd835, 0xdd3d, 0xd835,
0xdd4c, 0x0147, 0x2115,
0xff59, 0x0020, 0x0163,
0x4e47, 0xd835, 0xdd4f,
0xd835, 0xdce3
};
// UTF-16 bytes for "funny"
uint16_t funny[] = { 0x66, 0x75, 0x6e, 0x6e, 0x79 };
decancer_error_t error;
decancer_cured_t cured = decancer_cure_utf16(input, sizeof(input) / sizeof(uint16_t), DECANCER_OPTION_DEFAULT, &error);
if (cured == NULL) {
fprintf(stderr, "curing error: %.*s\n", (int)error.message_length, error.message);
return 1;
}
decancer_assert(decancer_contains_utf16(cured, funny, sizeof(funny) / sizeof(uint16_t)), "decancer_contains_utf16");
END:
decancer_cured_free(cured);
return ret;
}
Donations
If you want to support my eyes for manually looking at thousands of unicode characters, consider donating! ❤
Contributing
Please read CONTRIBUTING.md
for newbie contributors who want to contribute!
More Resourcesto explore the angular.
mail [email protected] to add your project or resources here 🔥.
- 1Create business apps like assembling blocks | ILLA Cloud
https://illacloud.com
Empower your team with AI Agent and advanced low-code tools to create business apps
- 2An operating system written in Rust
https://github.com/0x59616e/SteinsOS
An operating system written in Rust. Contribute to 0x59616e/SteinsOS development by creating an account on GitHub.
- 3Minimalistic program launcher
https://github.com/j0ru/kickoff
Minimalistic program launcher. Contribute to j0ru/kickoff development by creating an account on GitHub.
- 4Workflow runs · rust-lang/rustup
https://github.com/rust-lang/rustup/actions
The Rust toolchain installer. Contribute to rust-lang/rustup development by creating an account on GitHub.
- 5A simple, modular, and fast framework for writing MEV bots in Rust.
https://github.com/paradigmxyz/artemis
A simple, modular, and fast framework for writing MEV bots in Rust. - paradigmxyz/artemis
- 6Workflow runs · sigp/lighthouse
https://github.com/sigp/lighthouse/actions
Ethereum consensus client in Rust. Contribute to sigp/lighthouse development by creating an account on GitHub.
- 7Developer environments you can take with you
https://github.com/flox/flox
Developer environments you can take with you. Contribute to flox/flox development by creating an account on GitHub.
- 8Unified Architecture - OPC Foundation
https://opcfoundation.org/about/opc-technologies/opc-ua/
The OPC Unified Architecture (UA), released in 2008, is a platform independent service-oriented architecture that integrates all the functionality of the individual OPC Classic specifications into one extensible framework. This multi-layered approach accomplishes the original design specification goals of: Functional equivalence: all COM OPC Classic specifications are mapped to UA Platform independence: from an embedded [...]
- 9MaidSafe
https://github.com/maidsafe
MaidSafe has 71 repositories available. Follow their code on GitHub.
- 10ttyperacer / terminal-typeracer · GitLab
https://gitlab.com/ttyperacer/terminal-typeracer
GitLab.com
- 11👾 Modern and minimalist pixel editor
https://github.com/cloudhead/rx
👾 Modern and minimalist pixel editor. Contribute to cloudhead/rx development by creating an account on GitHub.
- 12Encode and decode smart contract invocations
https://github.com/rust-ethereum/ethabi
Encode and decode smart contract invocations. Contribute to rust-ethereum/ethabi development by creating an account on GitHub.
- 13Executes commands in response to file modifications
https://github.com/watchexec/watchexec
Executes commands in response to file modifications - watchexec/watchexec
- 14A terminal IRC client
https://github.com/osa1/tiny
A terminal IRC client . Contribute to osa1/tiny development by creating an account on GitHub.
- 15💓 Today's Trending Values for EDM Production
https://github.com/sergree/whatbpm
💓 Today's Trending Values for EDM Production. Contribute to sergree/whatbpm development by creating an account on GitHub.
- 16A roguelike game in Rust
https://github.com/rsaarelm/magog
A roguelike game in Rust. Contribute to rsaarelm/magog development by creating an account on GitHub.
- 17Subspace Network reference implementation
https://github.com/autonomys/subspace
Subspace Network reference implementation. Contribute to autonomys/subspace development by creating an account on GitHub.
- 18Provides a single TUI-based registry for drm-free, wine and steam games on linux, accessed through a rofi launch menu.
https://github.com/nicohman/eidolon
Provides a single TUI-based registry for drm-free, wine and steam games on linux, accessed through a rofi launch menu. - nicohman/eidolon
- 19A lightweight, terminal-based application to view and query delimiter separated value formatted documents, such as CSV or TSV files.
https://github.com/shshemi/tabiew
A lightweight, terminal-based application to view and query delimiter separated value formatted documents, such as CSV or TSV files. - shshemi/tabiew
- 20A GB emulator that is written in Rust 🦀!
https://github.com/joamag/boytacean
A GB emulator that is written in Rust 🦀! Contribute to joamag/boytacean development by creating an account on GitHub.
- 21Graph-oriented live coding language and music/audio DSP library written in Rust
https://github.com/chaosprint/glicol
Graph-oriented live coding language and music/audio DSP library written in Rust - chaosprint/glicol
- 22Winter is coming... ❄️
https://github.com/wasmerio/winterjs
Winter is coming... ❄️. Contribute to wasmerio/winterjs development by creating an account on GitHub.
- 23A new kind of terminal
https://github.com/withoutboats/notty
A new kind of terminal. Contribute to withoutboats/notty development by creating an account on GitHub.
- 24A multi-protocol proxy server written in Rust (HTTP, HTTPS, SOCKS5, Vmess, Vless, Shadowsocks, Trojan, Snell)
https://github.com/cfal/shoes
A multi-protocol proxy server written in Rust (HTTP, HTTPS, SOCKS5, Vmess, Vless, Shadowsocks, Trojan, Snell) - cfal/shoes
- 25A stateless trustless Starknet light client in Rust 🦀
https://github.com/eigerco/beerus
A stateless trustless Starknet light client in Rust 🦀 - eigerco/beerus
- 26evm toolkit
https://github.com/quilt/etk
evm toolkit. Contribute to quilt/etk development by creating an account on GitHub.
- 27System76 Power Management
https://github.com/pop-os/system76-power/
System76 Power Management. Contribute to pop-os/system76-power development by creating an account on GitHub.
- 28Custom Ethereum vanity address generator made in Rust
https://github.com/Limeth/ethaddrgen
Custom Ethereum vanity address generator made in Rust - Limeth/ethaddrgen
- 29The Tor Project / Core / Arti · GitLab
https://gitlab.torproject.org/tpo/core/arti
An implementation of Tor, in Rust. (So far, it's a not-very-complete client. But watch this space!)
- 30Production
https://www.rust-lang.org/production
A language empowering everyone to build reliable and efficient software.
- 31Shun Sakai / qrtool · GitLab
https://gitlab.com/sorairolake/qrtool
The upstream is https://github.com/sorairolake/qrtool
- 32Roll your own tracker!
https://github.com/ignisda/ryot
Roll your own tracker! Contribute to IgnisDa/ryot development by creating an account on GitHub.
- 33Workflow runs · keep-starknet-strange/madara
https://github.com/keep-starknet-strange/madara/actions/workflows/test.yml
DEPRECATED in favor of https://github.com/madara-alliance/madara - Workflow runs · keep-starknet-strange/madara
- 34Compile and Test · Workflow runs · Linus-Mussmaecher/rucola
https://github.com/Linus-Mussmaecher/rucola/actions/workflows/continuous-testing.yml
Terminal-based markdown note manager. Contribute to Linus-Mussmaecher/rucola development by creating an account on GitHub.
- 35redox-os / redox · GitLab
https://gitlab.redox-os.org/redox-os/redox
Redox: A Rust Operating System
- 36Discover historic Miner Extractable Value (MEV) opportunities
https://github.com/flashbots/mev-inspect-rs
Discover historic Miner Extractable Value (MEV) opportunities - flashbots/mev-inspect-rs
- 37CI · Workflow runs · GreptimeTeam/greptimedb
https://github.com/greptimeTeam/greptimedb/actions/workflows/develop.yml
An open-source, cloud-native, unified time series database for metrics, logs and events with SQL/PromQL supported. Available on GreptimeCloud. - CI · Workflow runs · GreptimeTeam/greptimedb
- 38Workflow runs · vrmiguel/bustd
https://github.com/vrmiguel/bustd/actions?query=branch%3Amaster
Process killer daemon for out-of-memory scenarios. Contribute to vrmiguel/bustd development by creating an account on GitHub.
- 39Performs distributed command execution, written in Rust w/ Tokio
https://github.com/mmstick/concurr
Performs distributed command execution, written in Rust w/ Tokio - mmstick/concurr
- 40A work-in-progress, open-source, multi-player city simulation game.
https://github.com/citybound/citybound
A work-in-progress, open-source, multi-player city simulation game. - citybound/citybound
- 41Test · Materialize
https://buildkite.com/materialize/test
Branches for Test: Run fast unit and integration tests
- 42A spotify daemon
https://github.com/Spotifyd/spotifyd
A spotify daemon. Contribute to Spotifyd/spotifyd development by creating an account on GitHub.
- 43A simple Git/Mercurial/PlasticSCM tui client based on keyboard shortcuts
https://github.com/vamolessa/verco
A simple Git/Mercurial/PlasticSCM tui client based on keyboard shortcuts - vamolessa/verco
- 44A more intuitive version of du in rust
https://github.com/bootandy/dust
A more intuitive version of du in rust. Contribute to bootandy/dust development by creating an account on GitHub.
- 45Yet another rust chip8 emulator
https://github.com/starrhorne/chip8-rust
Yet another rust chip8 emulator. Contribute to starrhorne/chip8-rust development by creating an account on GitHub.
- 46sniffnet/.github/workflows/rust.yml at main · GyulyVGC/sniffnet
https://github.com/GyulyVGC/sniffnet/blob/main/.github/workflows/rust.yml
Comfortably monitor your Internet traffic 🕵️♂️. Contribute to GyulyVGC/sniffnet development by creating an account on GitHub.
- 47Papercraft is a tool to unwrap 3D models.
https://github.com/rodrigorc/papercraft
Papercraft is a tool to unwrap 3D models. Contribute to rodrigorc/papercraft development by creating an account on GitHub.
- 48Workflow runs · denoland/deno
https://github.com/denoland/deno/actions
A modern runtime for JavaScript and TypeScript. Contribute to denoland/deno development by creating an account on GitHub.
- 49Veloren / veloren · GitLab
https://gitlab.com/veloren/veloren
Veloren is a multiplayer voxel RPG written in Rust. It is inspired by games such as Cube World, Legend of Zelda: Breath of the Wild, Dwarf Fortress and...
- 50Create book from markdown files. Like Gitbook but implemented in Rust
https://github.com/rust-lang/mdBook
Create book from markdown files. Like Gitbook but implemented in Rust - rust-lang/mdBook
- 51Polaris is a music streaming application, designed to let you enjoy your music collection from any computer or mobile device.
https://github.com/agersant/polaris
Polaris is a music streaming application, designed to let you enjoy your music collection from any computer or mobile device. - agersant/polaris
- 52Arbitrary-precision unit-aware calculator
https://github.com/printfn/fend
Arbitrary-precision unit-aware calculator. Contribute to printfn/fend development by creating an account on GitHub.
- 53Pipelines · Veloren / veloren · GitLab
https://gitlab.com/veloren/veloren/-/pipelines
Veloren is a multiplayer voxel RPG written in Rust. It is inspired by games such as Cube World, Legend of Zelda: Breath of the Wild, Dwarf Fortress and...
- 54TCP connection hijacker, Rust rewrite of shijack
https://github.com/kpcyrd/rshijack
TCP connection hijacker, Rust rewrite of shijack. Contribute to kpcyrd/rshijack development by creating an account on GitHub.
- 55Track your time without being tracked
https://github.com/lakoliu/Furtherance
Track your time without being tracked. Contribute to lakoliu/Furtherance development by creating an account on GitHub.
- 56Workflow runs · hcavarsan/kftray
https://github.com/hcavarsan/kftray/actions
A cross-platform system tray application for managing multiple kubectl port-forward commands, with support for UDP and proxy connections through k8s clusters - Workflow runs · hcavarsan/kftray
- 57Convert your favorite images and wallpapers with your favorite color palettes/themes
https://github.com/doprz/dipc
Convert your favorite images and wallpapers with your favorite color palettes/themes - doprz/dipc
- 58Workflow runs · dandavison/delta
https://github.com/dandavison/delta//actions
A syntax-highlighting pager for git, diff, grep, and blame output - Workflow runs · dandavison/delta
- 59Simple and fast Web server
https://github.com/wyhaya/see
Simple and fast Web server. Contribute to wyhaya/see development by creating an account on GitHub.
- 60Procedural engine sound generator controlled via GUI or CLI
https://github.com/DasEtwas/enginesound
Procedural engine sound generator controlled via GUI or CLI - DasEtwas/enginesound
- 61An efficient re-implementation of Electrum Server in Rust
https://github.com/romanz/electrs
An efficient re-implementation of Electrum Server in Rust - romanz/electrs
- 62A fast data collector in Rust
https://github.com/awslabs/flowgger
A fast data collector in Rust. Contribute to awslabs/flowgger development by creating an account on GitHub.
- 63Workflow runs · wasmerio/wasmer
https://github.com/wasmerio/wasmer/actions
🚀 The leading Wasm Runtime supporting WASIX, WASI and Emscripten - Workflow runs · wasmerio/wasmer
- 64CI · Workflow runs · hinto-janai/festival
https://github.com/hinto-janai/festival/actions/workflows/ci.yml
Music player. Contribute to hinto-janai/festival development by creating an account on GitHub.
- 65QA · Workflow runs · lambdaclass/cairo-vm
https://github.com/lambdaclass/cairo-vm/actions/workflows/rust.yml
cairo-vm is a Rust implementation of the Cairo VM. Cairo (CPU Algebraic Intermediate Representation) is a programming language for writing provable programs, where one party can prove to another th...
- 66A terminal workspace with batteries included
https://github.com/zellij-org/zellij
A terminal workspace with batteries included. Contribute to zellij-org/zellij development by creating an account on GitHub.
- 67A simple tui to view & control docker containers
https://github.com/mrjackwills/oxker
A simple tui to view & control docker containers . Contribute to mrjackwills/oxker development by creating an account on GitHub.
- 68Coinbase pro client for Rust
https://github.com/inv2004/coinbase-pro-rs
Coinbase pro client for Rust. Contribute to inv2004/coinbase-pro-rs development by creating an account on GitHub.
- 69A Mastodon-compatible, ActivityPub-speaking server in Rust
https://github.com/rustodon/rustodon
A Mastodon-compatible, ActivityPub-speaking server in Rust - rustodon/rustodon
- 70Comfortably monitor your Internet traffic 🕵️♂️
https://github.com/GyulyVGC/sniffnet
Comfortably monitor your Internet traffic 🕵️♂️. Contribute to GyulyVGC/sniffnet development by creating an account on GitHub.
- 71Drill is an HTTP load testing application written in Rust
https://github.com/fcsonline/drill
Drill is an HTTP load testing application written in Rust - fcsonline/drill
- 72Deploy · Workflow runs · michelhe/rustboyadvance-ng
https://github.com/michelhe/rustboyadvance-ng/actions?query=workflow%3ADeploy
RustBoyAdvance-NG is a Nintendo™ Game Boy Advance emulator and debugger, written in the rust programming language. - Deploy · Workflow runs · michelhe/rustboyadvance-ng
- 73Diem’s mission is to build a trusted and innovative financial network that empowers people and businesses around the world.
https://github.com/diem/diem
Diem’s mission is to build a trusted and innovative financial network that empowers people and businesses around the world. - diem/diem
- 74A hardware-accelerated GPU terminal emulator focusing to run in desktops and browsers.
https://github.com/raphamorim/rio
A hardware-accelerated GPU terminal emulator focusing to run in desktops and browsers. - raphamorim/rio
- 75Music Player TUI written in Rust
https://github.com/tramhao/termusic
Music Player TUI written in Rust. Contribute to tramhao/termusic development by creating an account on GitHub.
- 76Rust-based platform for the Web
https://github.com/swc-project/swc
Rust-based platform for the Web. Contribute to swc-project/swc development by creating an account on GitHub.
- 77Workflow runs · EasyTier/EasyTier
https://github.com/EasyTier/EasyTier/actions/
A simple, decentralized mesh VPN with WireGuard support. - Workflow runs · EasyTier/EasyTier
- 78Neon: Serverless Postgres. We separated storage and compute to offer autoscaling, code-like database branching, and scale to zero.
https://github.com/neondatabase/neon
Neon: Serverless Postgres. We separated storage and compute to offer autoscaling, code-like database branching, and scale to zero. - neondatabase/neon
- 79Rust bindings for MDBX
https://github.com/vorot93/libmdbx-rs
Rust bindings for MDBX. Contribute to vorot93/libmdbx-rs development by creating an account on GitHub.
- 80Joystream Monorepo
https://github.com/Joystream/joystream
Joystream Monorepo. Contribute to Joystream/joystream development by creating an account on GitHub.
- 81Immutable Ordered Key-Value Database Engine
https://github.com/PumpkinDB/PumpkinDB
Immutable Ordered Key-Value Database Engine. Contribute to PumpkinDB/PumpkinDB development by creating an account on GitHub.
- 82Turn your smartphone into presentation remote controller
https://github.com/thewh1teagle/mobslide
Turn your smartphone into presentation remote controller - thewh1teagle/mobslide
- 83Modern applications with built-in automation
https://github.com/habitat-sh/habitat
Modern applications with built-in automation. Contribute to habitat-sh/habitat development by creating an account on GitHub.
- 84A Spotify player in the terminal with full feature parity
https://github.com/aome510/spotify-player
A Spotify player in the terminal with full feature parity - aome510/spotify-player
- 85Workflow runs · terminusdb/terminusdb-store
https://github.com/terminusdb/terminusdb-store/actions
a tokio-enabled data store for triple data. Contribute to terminusdb/terminusdb-store development by creating an account on GitHub.
- 86A hackable, minimal, fast TUI file explorer
https://github.com/sayanarijit/xplr
A hackable, minimal, fast TUI file explorer. Contribute to sayanarijit/xplr development by creating an account on GitHub.
- 87CI · Workflow runs · open-telemetry/opentelemetry-rust
https://github.com/open-telemetry/opentelemetry-rust/actions?query=workflow%3ACI+branch%3Amaster
The Rust OpenTelemetry implementation. Contribute to open-telemetry/opentelemetry-rust development by creating an account on GitHub.
- 88✨ Magical shell history
https://github.com/atuinsh/atuin
✨ Magical shell history. Contribute to atuinsh/atuin development by creating an account on GitHub.
- 89A fast duplicate file finder
https://github.com/darakian/ddh
A fast duplicate file finder. Contribute to darakian/ddh development by creating an account on GitHub.
- 90interBTC: Bitcoin Anywhere
https://github.com/interlay/interbtc
interBTC: Bitcoin Anywhere. Contribute to interlay/interbtc development by creating an account on GitHub.
- 91ci · Workflow runs · nix-community/nix-melt
https://github.com/nix-community/nix-melt/actions/workflows/ci.yml
A ranger-like flake.lock viewer [maintainer=@figsoda] - ci · Workflow runs · nix-community/nix-melt
- 92A Rust implementation of BIP-0039
https://github.com/infincia/bip39-rs
A Rust implementation of BIP-0039 . Contribute to infincia/bip39-rs development by creating an account on GitHub.
- 93The only _real_ 2FA MFA WireGuard Enterprise VPN with build-in SSO, hardware keys management and more!
https://github.com/defguard/defguard
The only _real_ 2FA MFA WireGuard Enterprise VPN with build-in SSO, hardware keys management and more! - DefGuard/defguard
- 94Connect your local process and your cloud environment, and run local code in cloud conditions.
https://github.com/metalbear-co/mirrord
Connect your local process and your cloud environment, and run local code in cloud conditions. - metalbear-co/mirrord
- 95In-memory database inspired by erlang mnesia
https://github.com/Rustixir/darkbird
In-memory database inspired by erlang mnesia. Contribute to Rustixir/darkbird development by creating an account on GitHub.
- 96Mirror of https://gitlab.com/mmstick/tv-renamer
https://github.com/mmstick/tv-renamer
Mirror of https://gitlab.com/mmstick/tv-renamer. Contribute to mmstick/tv-renamer development by creating an account on GitHub.
- 97The Nervos CKB is a public permissionless blockchain, and the layer 1 of Nervos network.
https://github.com/nervosnetwork/ckb
The Nervos CKB is a public permissionless blockchain, and the layer 1 of Nervos network. - nervosnetwork/ckb
- 98Trustworthy, encrypted, command-line TOTP/HOTP authenticator app with import functionality.
https://github.com/replydev/cotp
Trustworthy, encrypted, command-line TOTP/HOTP authenticator app with import functionality. - replydev/cotp
- 99OpenID Connect Single Sign-On Identity & Access Management
https://github.com/sebadob/rauthy
OpenID Connect Single Sign-On Identity & Access Management - sebadob/rauthy
- 100The home for Hyperlane core contracts, sdk packages, and other infrastructure
https://github.com/hyperlane-xyz/hyperlane-monorepo
The home for Hyperlane core contracts, sdk packages, and other infrastructure - hyperlane-xyz/hyperlane-monorepo
- 101Rust Code Completion utility
https://github.com/racer-rust/racer
Rust Code Completion utility. Contribute to racer-rust/racer development by creating an account on GitHub.
- 102Releases · ShadoySV/work-break
https://github.com/ShadoySV/work-break/releases
Work-break balancer for Windows / MacOS / Linux desktops - ShadoySV/work-break
- 103kytan: High Performance Peer-to-Peer VPN in Rust
https://github.com/changlan/kytan
kytan: High Performance Peer-to-Peer VPN in Rust. Contribute to changlan/kytan development by creating an account on GitHub.
- 104Pinepods is a complete podcast management system and allows you to play, download, and keep track of podcasts you enjoy. All self hosted and enjoyed on your own server!
https://github.com/madeofpendletonwool/PinePods
Pinepods is a complete podcast management system and allows you to play, download, and keep track of podcasts you enjoy. All self hosted and enjoyed on your own server! - madeofpendletonwool/PinePods
- 105Intel 8080 cpu emulator by Rust
https://github.com/mohanson/i8080
Intel 8080 cpu emulator by Rust. Contribute to mohanson/i8080 development by creating an account on GitHub.
- 106SQLSync is a collaborative offline-first wrapper around SQLite. It is designed to synchronize web application state between users, devices, and the edge.
https://github.com/orbitinghail/sqlsync
SQLSync is a collaborative offline-first wrapper around SQLite. It is designed to synchronize web application state between users, devices, and the edge. - orbitinghail/sqlsync
- 107interative assembly shell written in rust
https://github.com/cch123/asm-cli-rust
interative assembly shell written in rust. Contribute to cch123/asm-cli-rust development by creating an account on GitHub.
- 108A library for building fast, reliable and evolvable network services.
https://github.com/cloudflare/pingora
A library for building fast, reliable and evolvable network services. - cloudflare/pingora
- 109A MITM Proxy 🧑💻! Toolkit for HTTP/1, HTTP/2, and WebSockets with SSL/TLS Capabilities. Learning Project.
https://github.com/emanuele-em/proxelar
A MITM Proxy 🧑💻! Toolkit for HTTP/1, HTTP/2, and WebSockets with SSL/TLS Capabilities. Learning Project. - emanuele-em/proxelar
- 110A high-performance observability data pipeline.
https://github.com/vectordotdev/vector
A high-performance observability data pipeline. Contribute to vectordotdev/vector development by creating an account on GitHub.
- 111Scientific calculator with math syntax that supports user-defined variables and functions, complex numbers, and estimation of derivatives and integrals
https://github.com/PaddiM8/kalker
Scientific calculator with math syntax that supports user-defined variables and functions, complex numbers, and estimation of derivatives and integrals - PaddiM8/kalker
- 112🚀 The leading Wasm Runtime supporting WASIX, WASI and Emscripten
https://github.com/wasmerio/wasmer
🚀 The leading Wasm Runtime supporting WASIX, WASI and Emscripten - wasmerio/wasmer
- 113🌲 Rust Filecoin Node Implementation
https://github.com/ChainSafe/forest
🌲 Rust Filecoin Node Implementation. Contribute to ChainSafe/forest development by creating an account on GitHub.
- 114Generate Nix packages from URLs with hash prefetching, dependency inference, license detection, and more [maintainer=@figsoda]
https://github.com/nix-community/nix-init
Generate Nix packages from URLs with hash prefetching, dependency inference, license detection, and more [maintainer=@figsoda] - nix-community/nix-init
- 115Rust crate to implement a counterpart to the PBRT book's (3rd edition) C++ code. See also https://www.rs-pbrt.org/about ...
https://github.com/wahn/rs_pbrt
Rust crate to implement a counterpart to the PBRT book's (3rd edition) C++ code. See also https://www.rs-pbrt.org/about ... - wahn/rs_pbrt
- 116Full fake REST API generator written with Rust
https://github.com/serayuzgur/weld
Full fake REST API generator written with Rust. Contribute to serayuzgur/weld development by creating an account on GitHub.
- 117HD wallet BIP-32 related key derivation utilities.
https://github.com/jjyr/hdwallet
HD wallet BIP-32 related key derivation utilities. - jjyr/hdwallet
- 118suckit/.github/workflows/build_and_test.yml at master · Skallwar/suckit
https://github.com/Skallwar/suckit/blob/master/.github/workflows/build_and_test.yml
Suck the InTernet. Contribute to Skallwar/suckit development by creating an account on GitHub.
- 119Servo, the embeddable, independent, memory-safe, modular, parallel web rendering engine
https://github.com/servo/servo
Servo, the embeddable, independent, memory-safe, modular, parallel web rendering engine - servo/servo
- 120A GPU-accelerated cross-platform terminal emulator and multiplexer written by @wez and implemented in Rust
https://github.com/wez/wezterm
A GPU-accelerated cross-platform terminal emulator and multiplexer written by @wez and implemented in Rust - wez/wezterm
- 121Foundry is a blazing fast, portable and modular toolkit for Ethereum application development written in Rust.
https://github.com/foundry-rs/foundry
Foundry is a blazing fast, portable and modular toolkit for Ethereum application development written in Rust. - foundry-rs/foundry
- 122Efficient Duplicate File Finder
https://github.com/pkolaczk/fclones
Efficient Duplicate File Finder. Contribute to pkolaczk/fclones development by creating an account on GitHub.
- 123Snake implemented in rust.
https://github.com/maras-archive/rsnake
Snake implemented in rust. Contribute to maras-archive/rsnake development by creating an account on GitHub.
- 124A simple, decentralized mesh VPN with WireGuard support.
https://github.com/EasyTier/EasyTier
A simple, decentralized mesh VPN with WireGuard support. - EasyTier/EasyTier
- 125DEPRECATED in favor of https://github.com/madara-alliance/madara
https://github.com/keep-starknet-strange/madara
DEPRECATED in favor of https://github.com/madara-alliance/madara - keep-starknet-strange/madara
- 126A purpose-built proxy for the Linkerd service mesh. Written in Rust.
https://github.com/linkerd/linkerd2-proxy
A purpose-built proxy for the Linkerd service mesh. Written in Rust. - linkerd/linkerd2-proxy
- 127cairo-vm is a Rust implementation of the Cairo VM. Cairo (CPU Algebraic Intermediate Representation) is a programming language for writing provable programs, where one party can prove to another that a certain computation was executed correctly without the need for this party to re-execute the same program.
https://github.com/lambdaclass/cairo-vm
cairo-vm is a Rust implementation of the Cairo VM. Cairo (CPU Algebraic Intermediate Representation) is a programming language for writing provable programs, where one party can prove to another th...
- 128N64 emulator written in Rust
https://github.com/gopher64/gopher64
N64 emulator written in Rust. Contribute to gopher64/gopher64 development by creating an account on GitHub.
- 129Workflow runs · qdrant/qdrant
https://github.com/qdrant/qdrant/actions
Qdrant - High-performance, massive-scale Vector Database for the next generation of AI. Also available in the cloud https://cloud.qdrant.io/ - Workflow runs · qdrant/qdrant
- 130Full featured Cross-platform GameBoy emulator by Rust. Forever boys!.
https://github.com/mohanson/gameboy
Full featured Cross-platform GameBoy emulator by Rust. Forever boys!. - mohanson/gameboy
- 131Open source close combat inspired game
https://github.com/buxx/OpenCombat
Open source close combat inspired game. Contribute to buxx/OpenCombat development by creating an account on GitHub.
- 132A Doom Renderer written in Rust.
https://github.com/cristicbz/rust-doom
A Doom Renderer written in Rust. Contribute to cristicbz/rust-doom development by creating an account on GitHub.
- 133A Nintendo DS emulator written in Rust for desktop devices and the web, with debugging features and a focus on accuracy
https://github.com/kelpsyberry/dust
A Nintendo DS emulator written in Rust for desktop devices and the web, with debugging features and a focus on accuracy - kelpsyberry/dust
- 134A new way to see and navigate directory trees : https://dystroy.org/broot
https://github.com/Canop/broot
A new way to see and navigate directory trees : https://dystroy.org/broot - Canop/broot
- 135RustBoyAdvance-NG is a Nintendo™ Game Boy Advance emulator and debugger, written in the rust programming language.
https://github.com/michelhe/rustboyadvance-ng
RustBoyAdvance-NG is a Nintendo™ Game Boy Advance emulator and debugger, written in the rust programming language. - michelhe/rustboyadvance-ng
- 136一个简单的游戏存档管理器
https://github.com/mcthesw/game-save-manager
一个简单的游戏存档管理器. Contribute to mcthesw/game-save-manager development by creating an account on GitHub.
- 137tauri · Workflow runs · mcthesw/game-save-manager
https://github.com/mcthesw/game-save-manager/actions/workflows/tauri.yml
一个简单的游戏存档管理器. Contribute to mcthesw/game-save-manager development by creating an account on GitHub.
- 138A cat(1) clone with wings.
https://github.com/sharkdp/bat
A cat(1) clone with wings. Contribute to sharkdp/bat development by creating an account on GitHub.
- 139A tokio-based modbus library
https://github.com/slowtec/tokio-modbus
A tokio-based modbus library. Contribute to slowtec/tokio-modbus development by creating an account on GitHub.
- 140🤖 The Modern Port Scanner 🤖
https://github.com/RustScan/RustScan
🤖 The Modern Port Scanner 🤖. Contribute to RustScan/RustScan development by creating an account on GitHub.
- 141CI · Workflow runs · 1History/1History
https://github.com/1History/1History/actions/workflows/CI.yml
All your history in one file. Contribute to 1History/1History development by creating an account on GitHub.
- 142It's my honor to drive you fucking fire faster, to have more time with your Family and Sunshine.This tool is for those who often want to search for a string Deeply into a directory in Recursive mode, but not with the great tools: grep, ack, ripgrep .........every thing should be Small, Thin, Fast, Lazy....without Think and Remember too much ...一个工具最大的价值不是它有多少功能,而是它能够让你以多快的速度达成所愿......
https://github.com/Lisprez/so_stupid_search
It's my honor to drive you fucking fire faster, to have more time with your Family and Sunshine.This tool is for those who often want to search for a string Deeply into a directory in Recursive...
- 143Modern, beginner-friendly 3D and 4D Rubik's cube simulator
https://github.com/HactarCE/Hyperspeedcube
Modern, beginner-friendly 3D and 4D Rubik's cube simulator - HactarCE/Hyperspeedcube
- 144An implementation of Sokoban in Rust
https://github.com/swatteau/sokoban-rs
An implementation of Sokoban in Rust. Contribute to swatteau/sokoban-rs development by creating an account on GitHub.
- 145An open-source remote desktop application designed for self-hosting, as an alternative to TeamViewer.
https://github.com/rustdesk/rustdesk
An open-source remote desktop application designed for self-hosting, as an alternative to TeamViewer. - rustdesk/rustdesk
- 146A terminal-based text editor written in Rust
https://github.com/gchp/iota
A terminal-based text editor written in Rust. Contribute to gchp/iota development by creating an account on GitHub.
- 147(Mirror) S3-compatible object store for small self-hosted geo-distributed deployments. Main repo: https://git.deuxfleurs.fr/Deuxfleurs/garage
https://github.com/deuxfleurs-org/garage
(Mirror) S3-compatible object store for small self-hosted geo-distributed deployments. Main repo: https://git.deuxfleurs.fr/Deuxfleurs/garage - deuxfleurs-org/garage
- 148😠⚔️😈 A minimalistic 2D turn-based tactical game in Rust
https://github.com/ozkriff/zemeroth
😠⚔️😈 A minimalistic 2D turn-based tactical game in Rust - ozkriff/zemeroth
- 149Work-break balancer for Windows / MacOS / Linux desktops
https://github.com/ShadoySV/work-break
Work-break balancer for Windows / MacOS / Linux desktops - ShadoySV/work-break
- 150The Cloud Operational Data Store: use SQL to transform, deliver, and act on fast-changing data.
https://github.com/MaterializeInc/materialize
The Cloud Operational Data Store: use SQL to transform, deliver, and act on fast-changing data. - MaterializeInc/materialize
- 151A Rust library for working with Bitcoin SV
https://github.com/brentongunning/rust-sv
A Rust library for working with Bitcoin SV. Contribute to brentongunning/rust-sv development by creating an account on GitHub.
- 152CLI tool to help keep track of your Git repositories, written in Rust
https://github.com/nickgerace/gfold
CLI tool to help keep track of your Git repositories, written in Rust - nickgerace/gfold
- 153yet another youtube down loader (Git mirror)
https://github.com/dertuxmalwieder/yaydl
yet another youtube down loader (Git mirror). Contribute to dertuxmalwieder/yaydl development by creating an account on GitHub.
- 154𝗗𝗮𝘁𝗮, 𝗔𝗻𝗮𝗹𝘆𝘁𝗶𝗰𝘀 & 𝗔𝗜. Modern alternative to Snowflake. Cost-effective and simple for massive-scale analytics. https://databend.com
https://github.com/datafuselabs/databend
𝗗𝗮𝘁𝗮, 𝗔𝗻𝗮𝗹𝘆𝘁𝗶𝗰𝘀 & 𝗔𝗜. Modern alternative to Snowflake. Cost-effective and simple for massive-scale analytics. https://databend.com - datafuselabs/databend
- 155A kanban board for the terminal built with ❤️ in Rust
https://github.com/yashs662/rust_kanban
A kanban board for the terminal built with ❤️ in Rust - yashs662/rust_kanban
- 156rust · Workflow runs · yoav-lavi/melody
https://github.com/yoav-lavi/melody/actions/workflows/rust.yml
Melody is a language that compiles to regular expressions and aims to be more readable and maintainable - rust · Workflow runs · yoav-lavi/melody
- 157A simple, secure and modern file encryption tool (and Rust library) with small explicit keys, no config options, and UNIX-style composability.
https://github.com/str4d/rage
A simple, secure and modern file encryption tool (and Rust library) with small explicit keys, no config options, and UNIX-style composability. - str4d/rage
- 158Rust implementation of ErgoTree interpreter and wallet-related features
https://github.com/ergoplatform/sigma-rust
Rust implementation of ErgoTree interpreter and wallet-related features - ergoplatform/sigma-rust
- 159SQL as a Function for Rust
https://github.com/KipData/FnckSQL
SQL as a Function for Rust. Contribute to KipData/FnckSQL development by creating an account on GitHub.
- 160A clickwheel iPod emulator (WIP)
https://github.com/daniel5151/clicky
A clickwheel iPod emulator (WIP). Contribute to daniel5151/clicky development by creating an account on GitHub.
- 161GTK application for browsing and installing fonts from Google's font archive
https://github.com/mmstick/fontfinder
GTK application for browsing and installing fonts from Google's font archive - mmstick/fontfinder
- 162Workflow runs · extrawurst/gitui
https://github.com/extrawurst/gitui/actions
Blazing 💥 fast terminal-ui for git written in rust 🦀 - Workflow runs · extrawurst/gitui
- 163Build · Workflow runs · hrkfdn/ncspot
https://github.com/hrkfdn/ncspot/actions?query=workflow%3ABuild
Cross-platform ncurses Spotify client written in Rust, inspired by ncmpc and the likes. - Build · Workflow runs · hrkfdn/ncspot
- 164Rust client to Opensea's APIs and Ethereum smart contracts
https://github.com/gakonst/opensea-rs
Rust client to Opensea's APIs and Ethereum smart contracts - gakonst/opensea-rs
- 165Process killer daemon for out-of-memory scenarios
https://github.com/vrmiguel/bustd
Process killer daemon for out-of-memory scenarios. Contribute to vrmiguel/bustd development by creating an account on GitHub.
- 166Modern protobuf package management
https://github.com/helsing-ai/buffrs
Modern protobuf package management. Contribute to helsing-ai/buffrs development by creating an account on GitHub.
- 167Main · Workflow runs · mtkennerly/ludusavi
https://github.com/mtkennerly/ludusavi/actions/workflows/main.yaml
Backup tool for PC game saves. Contribute to mtkennerly/ludusavi development by creating an account on GitHub.
- 168Git Query language is a SQL like language to perform queries on .git files with supports of most of SQL features such as grouping, ordering and aggregations functions
https://github.com/amrdeveloper/gql
Git Query language is a SQL like language to perform queries on .git files with supports of most of SQL features such as grouping, ordering and aggregations functions - AmrDeveloper/GQL
- 169An OS kernel written in rust. Non POSIX
https://github.com/thepowersgang/rust_os
An OS kernel written in rust. Non POSIX. Contribute to thepowersgang/rust_os development by creating an account on GitHub.
- 170Experimental blockchain database
https://github.com/paritytech/parity-db
Experimental blockchain database. Contribute to paritytech/parity-db development by creating an account on GitHub.
- 171Development Build · Workflow runs · obhq/obliteration
https://github.com/obhq/obliteration/actions/workflows/main.yml
Experimental PS4 emulator written in Rust for Windows, macOS and Linux - Development Build · Workflow runs · obhq/obliteration
- 172Aspiring vim-like text editor
https://github.com/mathall/rim
Aspiring vim-like text editor. Contribute to mathall/rim development by creating an account on GitHub.
- 173A minimalistic ARP scan tool written in Rust for fast local network scans
https://github.com/kongbytes/arp-scan-rs
A minimalistic ARP scan tool written in Rust for fast local network scans - kongbytes/arp-scan-rs
- 174Inspektor is a protocol-aware proxy that is used to enforce access policies👮
https://github.com/inspektor-dev/inspektor
Inspektor is a protocol-aware proxy that is used to enforce access policies👮 - inspektor-dev/inspektor
- 175Workflow runs · ilai-deutel/kibi
https://github.com/ilai-deutel/kibi/actions?query=branch%3Amaster
A text editor in ≤1024 lines of code, written in Rust - Workflow runs · ilai-deutel/kibi
- 176A modern replacement for ps written in Rust
https://github.com/dalance/procs
A modern replacement for ps written in Rust. Contribute to dalance/procs development by creating an account on GitHub.
- 177Kata Containers is an open source project and community working to build a standard implementation of lightweight Virtual Machines (VMs) that feel and perform like containers, but provide the workload isolation and security advantages of VMs. https://katacontainers.io/
https://github.com/kata-containers/kata-containers
Kata Containers is an open source project and community working to build a standard implementation of lightweight Virtual Machines (VMs) that feel and perform like containers, but provide the workl...
- 178A faithful and open-source remake of Cave Story's engine written in Rust
https://github.com/doukutsu-rs/doukutsu-rs
A faithful and open-source remake of Cave Story's engine written in Rust - doukutsu-rs/doukutsu-rs
- 179A smarter cd command. Supports all major shells.
https://github.com/ajeetdsouza/zoxide/
A smarter cd command. Supports all major shells. Contribute to ajeetdsouza/zoxide development by creating an account on GitHub.
- 180Sketch and take handwritten notes.
https://github.com/flxzt/rnote
Sketch and take handwritten notes. Contribute to flxzt/rnote development by creating an account on GitHub.
- 181A post-modern modal text editor.
https://github.com/helix-editor/helix
A post-modern modal text editor. Contribute to helix-editor/helix development by creating an account on GitHub.
- 182Workflow runs · j0ru/kickoff
https://github.com/j0ru/kickoff/actions
Minimalistic program launcher. Contribute to j0ru/kickoff development by creating an account on GitHub.
- 183Release · Workflow runs · lasantosr/intelli-shell
https://github.com/lasantosr/intelli-shell/actions/workflows/release.yml
Like IntelliSense, but for shells. Contribute to lasantosr/intelli-shell development by creating an account on GitHub.
- 184A high performance blockchain kernel for enterprise users.
https://github.com/citahub/cita
A high performance blockchain kernel for enterprise users. - citahub/cita
- 185WooriDB is a general purpose time serial database. It is schemaless, key-value storage and uses its own query syntax that is similar to SparQL.
https://github.com/naomijub/wooridb
WooriDB is a general purpose time serial database. It is schemaless, key-value storage and uses its own query syntax that is similar to SparQL. - naomijub/wooridb
- 186Generate Nix fetcher calls from repository URLs [maintainer=@figsoda]
https://github.com/nix-community/nurl
Generate Nix fetcher calls from repository URLs [maintainer=@figsoda] - nix-community/nurl
- 187The Phala Network Blockchain, pRuntime and the bridge.
https://github.com/Phala-Network/phala-blockchain
The Phala Network Blockchain, pRuntime and the bridge. - Phala-Network/phala-blockchain
- 188Release · Workflow runs · importantimport/hatsu
https://github.com/importantimport/hatsu/actions/workflows/release.yml
🩵 Self-hosted & Fully-automated ActivityPub Bridge for Static Sites. - Release · Workflow runs · importantimport/hatsu
- 189ranger-like terminal file manager written in Rust
https://github.com/kamiyaa/joshuto
ranger-like terminal file manager written in Rust. Contribute to kamiyaa/joshuto development by creating an account on GitHub.
- 190TUI for Telegram written in Rust 🦀
https://github.com/FedericoBruzzone/tgt
TUI for Telegram written in Rust 🦀. Contribute to FedericoBruzzone/tgt development by creating an account on GitHub.
- 191Test Rust · Workflow runs · ruffle-rs/ruffle
https://github.com/ruffle-rs/ruffle/actions/workflows/test_rust.yml
A Flash Player emulator written in Rust. Contribute to ruffle-rs/ruffle development by creating an account on GitHub.
- 192A command line tool that executes make target using fuzzy finder with preview window.
https://github.com/kyu08/fzf-make
A command line tool that executes make target using fuzzy finder with preview window. - kyu08/fzf-make
- 193Workflow runs · Nukesor/pueue
https://github.com/nukesor/pueue/actions
:stars: Manage your shell commands. Contribute to Nukesor/pueue development by creating an account on GitHub.
- 194Workflow runs · MASQ-Project/Node
https://github.com/MASQ-Project/Node/actions
MASQ combines the benefits of VPN and Tor technology to create a superior next-generation privacy software, where users are rewarded for supporting an uncensored global web. Users gain privacy and ...
- 195Minimal implementation of the Mimblewimble protocol.
https://github.com/mimblewimble/grin/
Minimal implementation of the Mimblewimble protocol. - mimblewimble/grin
- 196Simple http server in Rust (Windows/Mac/Linux)
https://github.com/TheWaWaR/simple-http-server
Simple http server in Rust (Windows/Mac/Linux). Contribute to TheWaWaR/simple-http-server development by creating an account on GitHub.
- 197Workflow runs · PaddiM8/kalker
https://github.com/PaddiM8/kalker/actions
Scientific calculator with math syntax that supports user-defined variables and functions, complex numbers, and estimation of derivatives and integrals - Workflow runs · PaddiM8/kalker
- 198A modern alternative to ls
https://github.com/eza-community/eza
A modern alternative to ls. Contribute to eza-community/eza development by creating an account on GitHub.
- 199test · Workflow runs · hickory-dns/hickory-dns
https://github.com/hickory-dns/hickory-dns/actions?query=workflow%3Atest
A Rust based DNS client, server, and resolver. Contribute to hickory-dns/hickory-dns development by creating an account on GitHub.
- 200All your history in one file.
https://github.com/1History/1History
All your history in one file. Contribute to 1History/1History development by creating an account on GitHub.
- 201MegaAntiCheat/client-backend
https://github.com/MegaAntiCheat/client-backend
Contribute to MegaAntiCheat/client-backend development by creating an account on GitHub.
- 202Workflow runs · containers/youki
https://github.com/containers/youki/actions
A container runtime written in Rust. Contribute to containers/youki development by creating an account on GitHub.
- 203The Parity Polkadot Blockchain SDK
https://github.com/paritytech/polkadot-sdk
The Parity Polkadot Blockchain SDK. Contribute to paritytech/polkadot-sdk development by creating an account on GitHub.
- 204A Linux script management CLI written in Rust
https://github.com/pier-cli/pier
A Linux script management CLI written in Rust. Contribute to pier-cli/pier development by creating an account on GitHub.
- 205Music player
https://github.com/hinto-janai/festival
Music player. Contribute to hinto-janai/festival development by creating an account on GitHub.
- 206Sui, a next-generation smart contract platform with high throughput, low latency, and an asset-oriented programming model powered by the Move programming language
https://github.com/MystenLabs/sui
Sui, a next-generation smart contract platform with high throughput, low latency, and an asset-oriented programming model powered by the Move programming language - MystenLabs/sui
- 207Workflow runs · Qrlew/qrlew
https://github.com/Qrlew/qrlew/actions
Contribute to Qrlew/qrlew development by creating an account on GitHub.
- 208RustZX CI · Workflow runs · rustzx/rustzx
https://github.com/rustzx/rustzx/actions/workflows/ci.yml
ZX Spectrum emulator written in Rust. Contribute to rustzx/rustzx development by creating an account on GitHub.
- 209Automated image compression for efficiently distributing images on the web.
https://github.com/imager-io/imager
Automated image compression for efficiently distributing images on the web. - imager-io/imager
- 210Test · Workflow runs · nikolassv/bartib
https://github.com/nikolassv/bartib/actions/workflows/test.yml
A simple timetracker for the command line. It saves a log of all tracked activities as a plaintext file and allows you to create flexible reports. - Test · Workflow runs · nikolassv/bartib
- 211background rust code check
https://github.com/Canop/bacon
background rust code check. Contribute to Canop/bacon development by creating an account on GitHub.
- 212Multi functional app to find duplicates, empty folders, similar images etc.
https://github.com/qarmin/czkawka
Multi functional app to find duplicates, empty folders, similar images etc. - qarmin/czkawka
- 213Create ctags/etags for a cargo project
https://github.com/dan-t/rusty-tags
Create ctags/etags for a cargo project. Contribute to dan-t/rusty-tags development by creating an account on GitHub.
- 214Theseus is a modern OS written from scratch in Rust that explores 𝐢𝐧𝐭𝐫𝐚𝐥𝐢𝐧𝐠𝐮𝐚𝐥 𝐝𝐞𝐬𝐢𝐠𝐧: closing the semantic gap between compiler and hardware by maximally leveraging the power of language safety and affine types. Theseus aims to shift OS responsibilities like resource management into the compiler.
https://github.com/theseus-os/Theseus
Theseus is a modern OS written from scratch in Rust that explores 𝐢𝐧𝐭𝐫𝐚𝐥𝐢𝐧𝐠𝐮𝐚𝐥 𝐝𝐞𝐬𝐢𝐠𝐧: closing the semantic gap between compiler and hardware by maximally leveraging the power of language safety an...
- 215Windows · Workflow runs · jqnatividad/qsv
https://github.com/jqnatividad/qsv/actions/workflows/rust-windows.yml
CSVs sliced, diced & analyzed. Contribute to jqnatividad/qsv development by creating an account on GitHub.
- 216🩵 Self-hosted & Fully-automated ActivityPub Bridge for Static Sites.
https://github.com/importantimport/hatsu
🩵 Self-hosted & Fully-automated ActivityPub Bridge for Static Sites. - importantimport/hatsu
- 217A container runtime written in Rust
https://github.com/containers/youki
A container runtime written in Rust. Contribute to containers/youki development by creating an account on GitHub.
- 218Secure and fast microVMs for serverless computing.
https://github.com/firecracker-microvm/firecracker
Secure and fast microVMs for serverless computing. - firecracker-microvm/firecracker
- 219A simple API client (postman like) in your terminal
https://github.com/Julien-cpsn/ATAC
A simple API client (postman like) in your terminal - Julien-cpsn/ATAC
- 220CI · Workflow runs · rksm/hot-lib-reloader-rs
https://github.com/rksm/hot-lib-reloader-rs/actions/workflows/ci.yml
Reload Rust code without app restarts. For faster feedback cycles. - CI · Workflow runs · rksm/hot-lib-reloader-rs
- 221Workflow runs · helix-editor/helix
https://github.com/helix-editor/helix/actions
A post-modern modal text editor. Contribute to helix-editor/helix development by creating an account on GitHub.
- 222Releases · yashs662/rust_kanban
https://github.com/yashs662/rust_kanban/releases
A kanban board for the terminal built with ❤️ in Rust - yashs662/rust_kanban
- 223Commodore 64 emulator written in Rust
https://github.com/kondrak/rust64
Commodore 64 emulator written in Rust. Contribute to kondrak/rust64 development by creating an account on GitHub.
- 224Continuous integration · Workflow runs · RustScan/RustScan
https://github.com/RustScan/RustScan/actions?query=workflow%3A%22Continuous+integration%22
🤖 The Modern Port Scanner 🤖. Contribute to RustScan/RustScan development by creating an account on GitHub.
- 225An open source headless CMS / real-time database. Powerful table editor, full-text search, and SDKs for JS / React / Svelte.
https://github.com/atomicdata-dev/atomic-server/
An open source headless CMS / real-time database. Powerful table editor, full-text search, and SDKs for JS / React / Svelte. - atomicdata-dev/atomic-server
- 226Workflow runs · ajeetdsouza/zoxide
https://github.com/ajeetdsouza/zoxide/actions
A smarter cd command. Supports all major shells. Contribute to ajeetdsouza/zoxide development by creating an account on GitHub.
- 227🚀 Deploy a modern low-code platform in 5 Seconds!
https://github.com/illacloud/illa
🚀 Deploy a modern low-code platform in 5 Seconds! Contribute to illacloud/illa development by creating an account on GitHub.
- 228Cross-platform Text Expander written in Rust
https://github.com/espanso/espanso
Cross-platform Text Expander written in Rust. Contribute to espanso/espanso development by creating an account on GitHub.
- 229Postgres for Search and Analytics
https://github.com/paradedb/paradedb/
Postgres for Search and Analytics. Contribute to paradedb/paradedb development by creating an account on GitHub.
- 230Interactive, file-level Time Machine-like tool for ZFS/btrfs/nilfs2 (and even Time Machine and Restic backups!)
https://github.com/kimono-koans/httm
Interactive, file-level Time Machine-like tool for ZFS/btrfs/nilfs2 (and even Time Machine and Restic backups!) - kimono-koans/httm
- 231Continuous SQL for event streams, database CDC, and time series. Perform streaming analytics, or build event-driven applications, real-time ETL pipelines, and feature stores in minutes. Unified streaming and batch processing. PostgreSQL compatible.
https://github.com/RisingWaveLabs/risingwave
Continuous SQL for event streams, database CDC, and time series. Perform streaming analytics, or build event-driven applications, real-time ETL pipelines, and feature stores in minutes. Unified str...
- 232Batch rename utility for developers
https://github.com/yaa110/nomino
Batch rename utility for developers. Contribute to yaa110/nomino development by creating an account on GitHub.
- 233ci · Workflow runs · nix-community/nurl
https://github.com/nix-community/nurl/actions/workflows/ci.yml
Generate Nix fetcher calls from repository URLs [maintainer=@figsoda] - ci · Workflow runs · nix-community/nurl
- 234Workflow runs · ouch-org/ouch
https://github.com/ouch-org/ouch/actions?query=branch%3Amaster
Painless compression and decompression in the terminal - Workflow runs · ouch-org/ouch
- 235Powerful database anonymizer with flexible rules. Written in Rust.
https://github.com/datanymizer/datanymizer
Powerful database anonymizer with flexible rules. Written in Rust. - datanymizer/datanymizer
- 236Build, operate, and evolve enterprise-grade GraphQL APIs easily with Hasura
https://hasura.io/
Unlock the speed and simplicity of GraphQL at any scale to effortlessly build, operate, and evolve enterprise-grade APIs across multiple domains.
- 237A Game Boy research project and emulator written in Rust
https://github.com/Gekkio/mooneye-gb
A Game Boy research project and emulator written in Rust - Gekkio/mooneye-gb
- 238A higher dimensional raytracing prototype with non-euclidean-like features
https://github.com/Limeth/euclider
A higher dimensional raytracing prototype with non-euclidean-like features - Limeth/euclider
- 239Qdrant - High-performance, massive-scale Vector Database for the next generation of AI. Also available in the cloud https://cloud.qdrant.io/
https://github.com/qdrant/qdrant
Qdrant - High-performance, massive-scale Vector Database for the next generation of AI. Also available in the cloud https://cloud.qdrant.io/ - qdrant/qdrant
- 240CI Linux · Workflow runs · FedericoBruzzone/tgt
https://github.com/FedericoBruzzone/tgt/actions/workflows/ci-linux.yml
TUI for Telegram written in Rust 🦀. Contribute to FedericoBruzzone/tgt development by creating an account on GitHub.
- 241CI · Workflow runs · dotenv-linter/dotenv-linter
https://github.com/dotenv-linter/dotenv-linter/actions?query=workflow%3ACI+branch%3Amaster
⚡️Lightning-fast linter for .env files. Written in Rust 🦀 - CI · Workflow runs · dotenv-linter/dotenv-linter
- 242Transcribe on your own!
https://github.com/thewh1teagle/vibe
Transcribe on your own! Contribute to thewh1teagle/vibe development by creating an account on GitHub.
- 243Small command-line JSON Log viewer
https://github.com/brocode/fblog
Small command-line JSON Log viewer. Contribute to brocode/fblog development by creating an account on GitHub.
- 244An SVG rendering library.
https://github.com/RazrFalcon/resvg
An SVG rendering library. Contribute to RazrFalcon/resvg development by creating an account on GitHub.
- 245Fast and multi-source CLI program for managing Minecraft mods and modpacks from Modrinth, CurseForge, and GitHub Releases
https://github.com/gorilla-devs/ferium
Fast and multi-source CLI program for managing Minecraft mods and modpacks from Modrinth, CurseForge, and GitHub Releases - gorilla-devs/ferium
- 246CI · Workflow runs · aaronriekenberg/rust-parallel
https://github.com/aaronriekenberg/rust-parallel/actions/workflows/CI.yml
Fast command line app in rust/tokio to run commands in parallel. Similar interface to GNU parallel or xargs plus useful features. Listed in Awesome Rust utilities. - CI · Workflow runs · aaronrie...
- 247🐀 A link aggregator and forum for the fediverse
https://github.com/LemmyNet/lemmy
🐀 A link aggregator and forum for the fediverse. Contribute to LemmyNet/lemmy development by creating an account on GitHub.
- 248An open source payments switch written in Rust to make payments fast, reliable and affordable
https://github.com/juspay/hyperswitch
An open source payments switch written in Rust to make payments fast, reliable and affordable - juspay/hyperswitch
- 249Yet another cross-platform graphical process/system monitor.
https://github.com/ClementTsang/bottom
Yet another cross-platform graphical process/system monitor. - ClementTsang/bottom
- 250rustic - fast, encrypted, and deduplicated backups powered by Rust
https://github.com/rustic-rs/rustic
rustic - fast, encrypted, and deduplicated backups powered by Rust - rustic-rs/rustic
- 251Client libraries for Tendermint/CometBFT in Rust!
https://github.com/informalsystems/tendermint-rs
Client libraries for Tendermint/CometBFT in Rust! Contribute to informalsystems/tendermint-rs development by creating an account on GitHub.
- 252A fast, simple, recursive content discovery tool written in Rust.
https://github.com/epi052/feroxbuster
A fast, simple, recursive content discovery tool written in Rust. - epi052/feroxbuster
- 253A powerful text templating tool.
https://github.com/replicadse/complate
A powerful text templating tool. Contribute to replicadse/complate development by creating an account on GitHub.
- 254Sexy fonts for the console
https://github.com/dominikwilkowski/cfonts
Sexy fonts for the console. Contribute to dominikwilkowski/cfonts development by creating an account on GitHub.
- 255An NES emulator written in Rust
https://github.com/koute/pinky
An NES emulator written in Rust. Contribute to koute/pinky development by creating an account on GitHub.
- 256Build · Workflow runs · raftario/licensor
https://github.com/raftario/licensor/actions/workflows/build.yml
write licenses to stdout. Contribute to raftario/licensor development by creating an account on GitHub.
- 257Workflow runs · eigerco/beerus
https://github.com/eigerco/beerus/actions/workflows/test.yml
A stateless trustless Starknet light client in Rust 🦀 - Workflow runs · eigerco/beerus
- 258A private network system that uses WireGuard under the hood.
https://github.com/tonarino/innernet
A private network system that uses WireGuard under the hood. - tonarino/innernet
- 259A hashdeep/md5tree (but much more) for media files
https://github.com/kimono-koans/dano
A hashdeep/md5tree (but much more) for media files - kimono-koans/dano
- 260Fast web applications through dynamic, partially-stateful dataflow
https://github.com/mit-pdos/noria
Fast web applications through dynamic, partially-stateful dataflow - mit-pdos/noria
- 261Redis re-implemented in Rust.
https://github.com/seppo0010/rsedis
Redis re-implemented in Rust. Contribute to seppo0010/rsedis development by creating an account on GitHub.
- 262BGP implemented in the Rust Programming Language
https://github.com/osrg/rustybgp
BGP implemented in the Rust Programming Language. Contribute to osrg/rustybgp development by creating an account on GitHub.
- 263a tokio-enabled data store for triple data
https://github.com/terminusdb/terminusdb-store
a tokio-enabled data store for triple data. Contribute to terminusdb/terminusdb-store development by creating an account on GitHub.
- 264Terminal disk space navigator 🔭
https://github.com/imsnif/diskonaut
Terminal disk space navigator 🔭. Contribute to imsnif/diskonaut development by creating an account on GitHub.
- 265Multithreaded PNG optimizer written in Rust
https://github.com/shssoichiro/oxipng
Multithreaded PNG optimizer written in Rust. Contribute to shssoichiro/oxipng development by creating an account on GitHub.
- 266A chess TUI implementation in rust 🦀
https://github.com/thomas-mauran/chess-tui
A chess TUI implementation in rust 🦀. Contribute to thomas-mauran/chess-tui development by creating an account on GitHub.
- 267Regression · Workflow runs · dalance/procs
https://github.com/dalance/procs/actions/workflows/regression.yml
A modern replacement for ps written in Rust. Contribute to dalance/procs development by creating an account on GitHub.
- 268CI · Workflow runs · espanso/espanso
https://github.com/espanso/espanso/actions/workflows/ci.yml
Cross-platform Text Expander written in Rust. Contribute to espanso/espanso development by creating an account on GitHub.
- 269Stop half-done APIs! Cherrybomb is a CLI tool that helps you avoid undefined user behaviour by auditing your API specifications, validating them and running API security tests.
https://github.com/blst-security/cherrybomb
Stop half-done APIs! Cherrybomb is a CLI tool that helps you avoid undefined user behaviour by auditing your API specifications, validating them and running API security tests. - blst-security/cher...
- 270An open-source, cloud-native, unified time series database for metrics, logs and events with SQL/PromQL supported. Available on GreptimeCloud.
https://github.com/grepTimeTeam/greptimedb/
An open-source, cloud-native, unified time series database for metrics, logs and events with SQL/PromQL supported. Available on GreptimeCloud. - GreptimeTeam/greptimedb
- 271Fast command line app in rust/tokio to run commands in parallel. Similar interface to GNU parallel or xargs plus useful features. Listed in Awesome Rust utilities.
https://github.com/aaronriekenberg/rust-parallel
Fast command line app in rust/tokio to run commands in parallel. Similar interface to GNU parallel or xargs plus useful features. Listed in Awesome Rust utilities. - aaronriekenberg/rust-parallel
- 272A scalable, distributed, collaborative, document-graph database, for the realtime web
https://github.com/surrealdb/surrealdb
A scalable, distributed, collaborative, document-graph database, for the realtime web - surrealdb/surrealdb
- 273Holo is a suite of routing protocols designed to support high-scale and automation-driven networks.
https://github.com/holo-routing/holo
Holo is a suite of routing protocols designed to support high-scale and automation-driven networks. - holo-routing/holo
- 274The current, performant & industrial strength version of Holochain on Rust.
https://github.com/holochain/holochain
The current, performant & industrial strength version of Holochain on Rust. - holochain/holochain
- 275Workflow runs · replicadse/complate
https://github.com/replicadse/complate/actions
A powerful text templating tool. Contribute to replicadse/complate development by creating an account on GitHub.
- 276Drop-in embedded database in Rust
https://github.com/vincent-herlemont/native_db
Drop-in embedded database in Rust. Contribute to vincent-herlemont/native_db development by creating an account on GitHub.
- 277Cross-platform clipboard API (text | image | rich text | html | files | monitoring changes) | 跨平台剪贴板 API(文本|图片|富文本|html|文件|监听变化) Windows,MacOS,Linux
https://github.com/ChurchTao/clipboard-rs
Cross-platform clipboard API (text | image | rich text | html | files | monitoring changes) | 跨平台剪贴板 API(文本|图片|富文本|html|文件|监听变化) Windows,MacOS,Linux - ChurchTao/clipboard-rs
- 278Utility that takes logs from anywhere and sends them to Telegram.
https://github.com/mxseev/logram
Utility that takes logs from anywhere and sends them to Telegram. - GitHub - mxseev/logram: Utility that takes logs from anywhere and sends them to Telegram.
- 279Workflow runs · rust-lang/mdBook
https://github.com/rust-lang/mdBook/actions
Create book from markdown files. Like Gitbook but implemented in Rust - Workflow runs · rust-lang/mdBook
- 280Terminal bandwidth utilization tool
https://github.com/imsnif/bandwhich
Terminal bandwidth utilization tool. Contribute to imsnif/bandwhich development by creating an account on GitHub.
- 281Vulkan path tracing with Rust
https://github.com/KaminariOS/rustracer
Vulkan path tracing with Rust. Contribute to KaminariOS/rustracer development by creating an account on GitHub.
- 282an experimental package manager for operating systems
https://github.com/lodosgroup/lpm
an experimental package manager for operating systems - lodosgroup/lpm
- 283A ranger-like flake.lock viewer [maintainer=@figsoda]
https://github.com/nix-community/nix-melt
A ranger-like flake.lock viewer [maintainer=@figsoda] - nix-community/nix-melt
- 284High performance and distributed KV store w/ REST API. 🦀
https://github.com/lucid-kv/lucid
High performance and distributed KV store w/ REST API. 🦀 - lucid-kv/lucid
- 285Configuration Management for Localhost / dotfiles
https://github.com/comtrya/comtrya
Configuration Management for Localhost / dotfiles. Contribute to comtrya/comtrya development by creating an account on GitHub.
- 286CICD · Workflow runs · envio-cli/envio
https://github.com/envio-cli/envio/actions/workflows/CICD.yml
Envio is a modern and secure command-line tool that simplifies the management of environment variables - CICD · Workflow runs · envio-cli/envio
- 287🚀 10x easier, 🚀 140x lower storage cost, 🚀 high performance, 🚀 petabyte scale - Elasticsearch/Splunk/Datadog alternative for 🚀 (logs, metrics, traces, RUM, Error tracking, Session replay).
https://github.com/openobserve/openobserve
🚀 10x easier, 🚀 140x lower storage cost, 🚀 high performance, 🚀 petabyte scale - Elasticsearch/Splunk/Datadog alternative for 🚀 (logs, metrics, traces, RUM, Error tracking, Session replay). - openo...
- 288Cross-platform ncurses Spotify client written in Rust, inspired by ncmpc and the likes.
https://github.com/hrkfdn/ncspot
Cross-platform ncurses Spotify client written in Rust, inspired by ncmpc and the likes. - hrkfdn/ncspot
- 289An efficient way to filter duplicate lines from input, à la uniq.
https://github.com/whitfin/runiq
An efficient way to filter duplicate lines from input, à la uniq. - GitHub - whitfin/runiq: An efficient way to filter duplicate lines from input, à la uniq.
- 290Complete Ethereum & Celo library and wallet implementation in Rust. https://docs.rs/ethers
https://github.com/gakonst/ethers-rs
Complete Ethereum & Celo library and wallet implementation in Rust. https://docs.rs/ethers - gakonst/ethers-rs
- 291Userspace WireGuard® Implementation in Rust
https://github.com/cloudflare/boringtun
Userspace WireGuard® Implementation in Rust. Contribute to cloudflare/boringtun development by creating an account on GitHub.
- 292An old-school bash-like Unix shell written in Rust
https://github.com/mitnk/cicada
An old-school bash-like Unix shell written in Rust - mitnk/cicada
- 293:stars: Manage your shell commands.
https://github.com/nukesor/pueue
:stars: Manage your shell commands. Contribute to Nukesor/pueue development by creating an account on GitHub.
- 294A rewrite of the GameMaker Classic engine runners with additional tooling
https://github.com/OpenGMK/OpenGMK
A rewrite of the GameMaker Classic engine runners with additional tooling - OpenGMK/OpenGMK
- 295Synapse BitTorrent Daemon
https://github.com/Luminarys/synapse
Synapse BitTorrent Daemon. Contribute to Luminarys/synapse development by creating an account on GitHub.
- 296Findex is a highly customizable application finder written in Rust and uses GTK3
https://github.com/mdgaziur/findex
Findex is a highly customizable application finder written in Rust and uses GTK3 - mdgaziur/findex
- 297⬡ Zone of Control is a hexagonal turn-based strategy game written in Rust. [DISCONTINUED]
https://github.com/ozkriff/zoc
⬡ Zone of Control is a hexagonal turn-based strategy game written in Rust. [DISCONTINUED] - GitHub - ozkriff/zoc: ⬡ Zone of Control is a hexagonal turn-based strategy game written in Rust. [DISCON...
- 298Check · Workflow runs · sstadick/crabz
https://github.com/sstadick/crabz/actions?query=workflow%3ACheck
Like pigz, but rust. Contribute to sstadick/crabz development by creating an account on GitHub.
- 299a Lightweight, Permanent JSON document database
https://github.com/dbpunk-labs/db3
a Lightweight, Permanent JSON document database. Contribute to dbpunk-labs/db3 development by creating an account on GitHub.
- 300UpVPN is the world's first Serverless VPN. The VPN app is available for macOS, Linux, Windows, and Android. The UpVPN service can also be used with any WireGuard compatible client using the Web Device feature.
https://github.com/upvpn/upvpn-app
UpVPN is the world's first Serverless VPN. The VPN app is available for macOS, Linux, Windows, and Android. The UpVPN service can also be used with any WireGuard compatible client using the Web...
- 301Distributed transactional key-value database, originally created to complement TiDB
https://github.com/tikv/tikv
Distributed transactional key-value database, originally created to complement TiDB - tikv/tikv
- 302Test Web · Workflow runs · ruffle-rs/ruffle
https://github.com/ruffle-rs/ruffle/actions/workflows/test_web.yml
A Flash Player emulator written in Rust. Contribute to ruffle-rs/ruffle development by creating an account on GitHub.
- 303🔭 A simple ray tracer in Rust 🦀
https://github.com/dps/rust-raytracer
🔭 A simple ray tracer in Rust 🦀. Contribute to dps/rust-raytracer development by creating an account on GitHub.
- 304An experimental HTTP load testing application written in Rust.
https://github.com/imjacobclark/Herd
An experimental HTTP load testing application written in Rust. - imjacobclark/Herd
- 305A Command Line OTP Authenticator application.
https://github.com/evansmurithi/cloak
A Command Line OTP Authenticator application. Contribute to evansmurithi/cloak development by creating an account on GitHub.
- 306Set up a modern rust+react web app by running one command.
https://github.com/Wulf/create-rust-app
Set up a modern rust+react web app by running one command. - GitHub - Wulf/create-rust-app: Set up a modern rust+react web app by running one command.
- 307workspace productivity booster
https://github.com/brocode/fw
workspace productivity booster. Contribute to brocode/fw development by creating an account on GitHub.
- 308👽 A wrapper around restic built in rust
https://github.com/alvaro17f/wrestic
👽 A wrapper around restic built in rust. Contribute to alvaro17f/wrestic development by creating an account on GitHub.
- 309build and test · Workflow runs · AFLplusplus/LibAFL
https://github.com/AFLplusplus/LibAFL/actions/workflows/build_and_test.yml
Advanced Fuzzing Library - Slot your Fuzzer together in Rust! Scales across cores and machines. For Windows, Android, MacOS, Linux, no_std, ... - build and test · Workflow runs · AFLplusplus/LibAFL
- 310A new approach to Emacs - Including TypeScript, Threading, Async I/O, and WebRender.
https://github.com/emacs-ng/emacs-ng
A new approach to Emacs - Including TypeScript, Threading, Async I/O, and WebRender. - GitHub - emacs-ng/emacs-ng: A new approach to Emacs - Including TypeScript, Threading, Async I/O, and WebRender.
- 311Bitcoin's layer2 smart contract network has already supported WASM and EVM, and is supporting MoveVM
https://github.com/chainx-org/ChainX
Bitcoin's layer2 smart contract network has already supported WASM and EVM, and is supporting MoveVM - GitHub - chainx-org/ChainX: Bitcoin's layer2 smart contract network has already suppo...
- 312Quake map renderer in Rust
https://github.com/Thinkofname/rust-quake
Quake map renderer in Rust. Contribute to Thinkofname/rust-quake development by creating an account on GitHub.
- 313Ethereum consensus client in Rust
https://github.com/sigp/lighthouse
Ethereum consensus client in Rust. Contribute to sigp/lighthouse development by creating an account on GitHub.
- 314⚡ Energy consumption metrology agent. Let "scaph" dive and bring back the metrics that will help you make your systems and applications more sustainable !
https://github.com/hubblo-org/scaphandre
⚡ Energy consumption metrology agent. Let "scaph" dive and bring back the metrics that will help you make your systems and applications more sustainable ! - hubblo-org/scaphandre
- 315A user- and resources-friendly signatures-based malware scanner
https://github.com/Raspirus/Raspirus
A user- and resources-friendly signatures-based malware scanner - Raspirus/Raspirus
- 316xargs + awk with pattern matching support. `ls *.bak | rargs -p '(.*)\.bak' mv {0} {1}`
https://github.com/lotabout/rargs
xargs + awk with pattern matching support. `ls *.bak | rargs -p '(.*)\.bak' mv {0} {1}` - lotabout/rargs
- 317Minimal mpd terminal client that aims to be simple yet highly configurable
https://github.com/figsoda/mmtc
Minimal mpd terminal client that aims to be simple yet highly configurable - figsoda/mmtc
- 318ZX Spectrum emulator written in Rust
https://github.com/rustzx/rustzx
ZX Spectrum emulator written in Rust. Contribute to rustzx/rustzx development by creating an account on GitHub.
- 319Workflow runs · shssoichiro/oxipng
https://github.com/shssoichiro/oxipng/actions?query=branch%3Amaster
Multithreaded PNG optimizer written in Rust. Contribute to shssoichiro/oxipng development by creating an account on GitHub.
- 320Angolmois BMS player, Rust edition
https://github.com/lifthrasiir/angolmois-rust
Angolmois BMS player, Rust edition. Contribute to lifthrasiir/angolmois-rust development by creating an account on GitHub.
- 321Convert your ascii diagram scribbles into happy little SVG
https://github.com/ivanceras/svgbob
Convert your ascii diagram scribbles into happy little SVG - ivanceras/svgbob
- 322Advanced Fuzzing Library - Slot your Fuzzer together in Rust! Scales across cores and machines. For Windows, Android, MacOS, Linux, no_std, ...
https://github.com/AFLplusplus/LibAFL
Advanced Fuzzing Library - Slot your Fuzzer together in Rust! Scales across cores and machines. For Windows, Android, MacOS, Linux, no_std, ... - AFLplusplus/LibAFL
- 323Linux AMDGPU Configuration Tool
https://github.com/ilya-zlobintsev/LACT
Linux AMDGPU Configuration Tool. Contribute to ilya-zlobintsev/LACT development by creating an account on GitHub.
- 324Test backend · Workflow runs · Raspirus/Raspirus
https://github.com/Raspirus/Raspirus/actions/workflows/testproject.yml
A user- and resources-friendly signatures-based malware scanner - Test backend · Workflow runs · Raspirus/Raspirus
- 325Workflow runs · comtrya/comtrya
https://github.com/comtrya/comtrya/actions
Configuration Management for Localhost / dotfiles. Contribute to comtrya/comtrya development by creating an account on GitHub.
- 326Envio is a modern and secure command-line tool that simplifies the management of environment variables
https://github.com/envio-cli/envio
Envio is a modern and secure command-line tool that simplifies the management of environment variables - envio-cli/envio
- 327Run multiple commands in parallel
https://github.com/pvolok/mprocs
Run multiple commands in parallel. Contribute to pvolok/mprocs development by creating an account on GitHub.
- 328Terminal-based markdown note manager.
https://github.com/Linus-Mussmaecher/rucola
Terminal-based markdown note manager. Contribute to Linus-Mussmaecher/rucola development by creating an account on GitHub.
- 329🌞 🦀 🌙 Weather companion for the terminal. Rust app.
https://github.com/ttytm/wthrr-the-weathercrab
🌞 🦀 🌙 Weather companion for the terminal. Rust app. - GitHub - ttytm/wthrr-the-weathercrab: 🌞 🦀 🌙 Weather companion for the terminal. Rust app.
- 330Like pigz, but rust
https://github.com/sstadick/crabz
Like pigz, but rust. Contribute to sstadick/crabz development by creating an account on GitHub.
- 331A portable embedded database using Arrow.
https://github.com/tonbo-io/tonbo
A portable embedded database using Arrow. Contribute to tonbo-io/tonbo development by creating an account on GitHub.
- 332Terminal Network scanner & diagnostic tool with modern TUI
https://github.com/Chleba/netscanner
Terminal Network scanner & diagnostic tool with modern TUI - Chleba/netscanner
- 333Workflow runs · datafuselabs/databend
https://github.com/datafuselabs/databend/actions/workflows/databend-release.yml
𝗗𝗮𝘁𝗮, 𝗔𝗻𝗮𝗹𝘆𝘁𝗶𝗰𝘀 & 𝗔𝗜. Modern alternative to Snowflake. Cost-effective and simple for massive-scale analytics. https://databend.com - Workflow runs · datafuselabs/databend
- 334Secure multithreaded packet sniffer
https://github.com/kpcyrd/sniffglue
Secure multithreaded packet sniffer. Contribute to kpcyrd/sniffglue development by creating an account on GitHub.
- 335⚡A CLI tool for code structural search, lint and rewriting. Written in Rust
https://github.com/ast-grep/ast-grep
⚡A CLI tool for code structural search, lint and rewriting. Written in Rust - ast-grep/ast-grep
- 336A Gameboy Emulator in Rust
https://github.com/mvdnes/rboy
A Gameboy Emulator in Rust. Contribute to mvdnes/rboy development by creating an account on GitHub.
- 337Lucid · Workflow runs · lucid-kv/lucid
https://github.com/lucid-kv/lucid/actions?workflow=Lucid
High performance and distributed KV store w/ REST API. 🦀 - Lucid · Workflow runs · lucid-kv/lucid
- 338Qrlew/qrlew
https://github.com/Qrlew/qrlew
Contribute to Qrlew/qrlew development by creating an account on GitHub.
- 339Distributed SQL database in Rust, written as an educational project
https://github.com/erikgrinaker/toydb
Distributed SQL database in Rust, written as an educational project - erikgrinaker/toydb
- 340CI macOS · Workflow runs · FedericoBruzzone/tgt
https://github.com/FedericoBruzzone/tgt/actions/workflows/ci-macos.yml
TUI for Telegram written in Rust 🦀. Contribute to FedericoBruzzone/tgt development by creating an account on GitHub.
- 341CI · Workflow runs · quickwit-oss/quickwit
https://github.com/quickwit-oss/quickwit/actions?query=workflow%3ACI
Cloud-native search engine for observability. An open-source alternative to Datadog, Elasticsearch, Loki, and Tempo. - CI · Workflow runs · quickwit-oss/quickwit
- 342Backend service to build customer facing dashboards 10x faster. Written in Rust.
https://github.com/FrolicOrg/Frolic
Backend service to build customer facing dashboards 10x faster. Written in Rust. - frolicorg/frolic
- 343Workflow runs · entropic-security/xgadget
https://github.com/entropic-security/xgadget/actions
Fast, parallel, cross-variant ROP/JOP gadget search for x86/x64 binaries. - Workflow runs · entropic-security/xgadget
- 344A lightweight blazingly fast file watcher.
https://github.com/cristianoliveira/funzzy
A lightweight blazingly fast file watcher. Contribute to cristianoliveira/funzzy development by creating an account on GitHub.
- 345CI Windows · Workflow runs · FedericoBruzzone/tgt
https://github.com/FedericoBruzzone/tgt/actions/workflows/ci-windows.yml
TUI for Telegram written in Rust 🦀. Contribute to FedericoBruzzone/tgt development by creating an account on GitHub.
- 346Cleans dependencies and build artifacts from your projects.
https://github.com/tbillington/kondo
Cleans dependencies and build artifacts from your projects. - tbillington/kondo
- 347Skia based 2d graphics vue rendering library. It is based on Rust to implement software rasterization to perform rendering. 基于 Skia 的 2D 图形 Vue 渲染库 —— 使用 Rust 语言实现纯软件光栅化
https://github.com/rustq/vue-skia
Skia based 2d graphics vue rendering library. It is based on Rust to implement software rasterization to perform rendering. 基于 Skia 的 2D 图形 Vue 渲染库 —— 使用 Rust 语言实现纯软件光栅化 - rustq/vue-skia
- 348Vagga is a containerization tool without daemons
https://github.com/tailhook/vagga
Vagga is a containerization tool without daemons. Contribute to tailhook/vagga development by creating an account on GitHub.
- 349Scriptable network authentication cracker (formerly `badtouch`)
https://github.com/kpcyrd/authoscope
Scriptable network authentication cracker (formerly `badtouch`) - kpcyrd/authoscope
- 350Mk48.io ship combat game
https://github.com/SoftbearStudios/mk48
Mk48.io ship combat game. Contribute to SoftbearStudios/mk48 development by creating an account on GitHub.
- 351A transactional, relational-graph-vector database that uses Datalog for query. The hippocampus for AI!
https://github.com/cozodb/cozo
A transactional, relational-graph-vector database that uses Datalog for query. The hippocampus for AI! - cozodb/cozo
- 352A highly modular Bitcoin Lightning library written in Rust. It's rust-lightning, not Rusty's Lightning!
https://github.com/lightningdevkit/rust-lightning
A highly modular Bitcoin Lightning library written in Rust. It's rust-lightning, not Rusty's Lightning! - lightningdevkit/rust-lightning
- 353A text editor in ≤1024 lines of code, written in Rust
https://github.com/ilai-deutel/kibi
A text editor in ≤1024 lines of code, written in Rust - ilai-deutel/kibi
- 354Fuzzy Finder in rust!
https://github.com/lotabout/skim
Fuzzy Finder in rust! Contribute to lotabout/skim development by creating an account on GitHub.
- 355merge · Workflow runs · nickgerace/gfold
https://github.com/nickgerace/gfold/actions?query=workflow%3Amerge+branch%3Amain
CLI tool to help keep track of your Git repositories, written in Rust - merge · Workflow runs · nickgerace/gfold
- 356Workflow runs · holochain/holochain
https://github.com/holochain/holochain/actions/workflows/check_run_detect_release_pr_failure.yml
The current, performant & industrial strength version of Holochain on Rust. - Workflow runs · holochain/holochain
- 357Workflow runs · atomicdata-dev/atomic-server
https://github.com/atomicdata-dev/atomic-server/actions/workflows/docker.yml
An open source headless CMS / real-time database. Powerful table editor, full-text search, and SDKs for JS / React / Svelte. - Workflow runs · atomicdata-dev/atomic-server
- 358A WebAssembly CHIP-8 emulator written with Rust
https://github.com/ColinEberhardt/wasm-rust-chip8
A WebAssembly CHIP-8 emulator written with Rust. Contribute to ColinEberhardt/wasm-rust-chip8 development by creating an account on GitHub.
- 359Zcash - Internet Money
https://github.com/zcash/zcash
Zcash - Internet Money. Contribute to zcash/zcash development by creating an account on GitHub.
- 360A toy ray tracer in Rust
https://github.com/Twinklebear/tray_rust
A toy ray tracer in Rust. Contribute to Twinklebear/tray_rust development by creating an account on GitHub.
- 361Extensible open world rogue like game with pixel art. Players can explore the wilderness and ruins.
https://github.com/garkimasera/rusted-ruins
Extensible open world rogue like game with pixel art. Players can explore the wilderness and ruins. - garkimasera/rusted-ruins
- 362Semi-automatic OSINT framework and package manager
https://github.com/kpcyrd/sn0int
Semi-automatic OSINT framework and package manager - kpcyrd/sn0int
- 363Build · Workflow runs · cozodb/cozo
https://github.com/cozodb/cozo/actions/workflows/build.yml
A transactional, relational-graph-vector database that uses Datalog for query. The hippocampus for AI! - Build · Workflow runs · cozodb/cozo
- 364ci · Workflow runs · figsoda/mmtc
https://github.com/figsoda/mmtc/actions/workflows/ci.yml
Minimal mpd terminal client that aims to be simple yet highly configurable - ci · Workflow runs · figsoda/mmtc
- 365Rust · Workflow runs · spacejam/sled
https://github.com/spacejam/sled/actions?workflow=Rust
the champagne of beta embedded databases. Contribute to spacejam/sled development by creating an account on GitHub.
- 366like ~~grep~~ UBER, but for binaries
https://github.com/m4b/bingrep
like ~~grep~~ UBER, but for binaries. Contribute to m4b/bingrep development by creating an account on GitHub.
- 367Experimental PS4 emulator written in Rust for Windows, macOS and Linux
https://github.com/obhq/obliteration
Experimental PS4 emulator written in Rust for Windows, macOS and Linux - obhq/obliteration
- 368Fast, parallel, cross-variant ROP/JOP gadget search for x86/x64 binaries.
https://github.com/entropic-security/xgadget
Fast, parallel, cross-variant ROP/JOP gadget search for x86/x64 binaries. - entropic-security/xgadget
- 369IBC Relayer in Rust
https://github.com/informalsystems/hermes
IBC Relayer in Rust. Contribute to informalsystems/hermes development by creating an account on GitHub.
- 370Build · Workflow runs · dani-garcia/vaultwarden
https://github.com/dani-garcia/vaultwarden/actions/workflows/build.yml
Unofficial Bitwarden compatible server written in Rust, formerly known as bitwarden_rs - Build · Workflow runs · dani-garcia/vaultwarden
- 371Cloud-native search engine for observability. An open-source alternative to Datadog, Elasticsearch, Loki, and Tempo.
https://github.com/quickwit-oss/quickwit
Cloud-native search engine for observability. An open-source alternative to Datadog, Elasticsearch, Loki, and Tempo. - quickwit-oss/quickwit
- 372Workflow runs · orbitinghail/sqlsync
https://github.com/orbitinghail/sqlsync/actions?query=branch%3Amain
SQLSync is a collaborative offline-first wrapper around SQLite. It is designed to synchronize web application state between users, devices, and the edge. - Workflow runs · orbitinghail/sqlsync
- 373Blazing 💥 fast terminal-ui for git written in rust 🦀
https://github.com/extrawurst/gitui
Blazing 💥 fast terminal-ui for git written in rust 🦀 - extrawurst/gitui
- 374A frontend to Assets purchased on Epic Games Store
https://github.com/AchetaGames/Epic-Asset-Manager
A frontend to Assets purchased on Epic Games Store - AchetaGames/Epic-Asset-Manager
- 375A modern runtime for JavaScript and TypeScript.
https://github.com/denoland/deno
A modern runtime for JavaScript and TypeScript. Contribute to denoland/deno development by creating an account on GitHub.
- 376A lightweight and distributed task scheduling platform written in rust. (一个轻量的分布式的任务调度平台通过rust编写)
https://github.com/BinChengZhao/delicate
A lightweight and distributed task scheduling platform written in rust. (一个轻量的分布式的任务调度平台通过rust编写) - BinChengZhao/delicate
- 377Workflow runs · orhun/git-cliff
https://github.com/orhun/git-cliff/actions
A highly customizable Changelog Generator that follows Conventional Commit specifications ⛰️ - Workflow runs · orhun/git-cliff
- 378NES emulator written in Rust
https://github.com/pcwalton/sprocketnes
NES emulator written in Rust. Contribute to pcwalton/sprocketnes development by creating an account on GitHub.
- 379Create new page · PistonDevelopers/piston Wiki
https://github.com/PistonDevelopers/piston/wiki/Games-Made-With-Piston.
A modular game engine written in Rust. Contribute to PistonDevelopers/piston development by creating an account on GitHub.
- 380A simple password manager written in Rust
https://github.com/cortex/ripasso/
A simple password manager written in Rust. Contribute to cortex/ripasso development by creating an account on GitHub.
- 381Workflow runs · surrealdb/surrealdb
https://github.com/surrealdb/surrealdb/actions
A scalable, distributed, collaborative, document-graph database, for the realtime web - Workflow runs · surrealdb/surrealdb
- 382Workflow runs · lsd-rs/lsd
https://github.com/lsd-rs/lsd/actions
The next gen ls command. Contribute to lsd-rs/lsd development by creating an account on GitHub.
- 383A client and server implementation of the OPC UA specification written in Rust
https://github.com/locka99/opcua
A client and server implementation of the OPC UA specification written in Rust - locka99/opcua
- 384Workflow runs · ClementTsang/bottom
https://github.com/ClementTsang/bottom/actions?query=branch%3Amaster
Yet another cross-platform graphical process/system monitor. - Workflow runs · ClementTsang/bottom
- 385Run tests · Workflow runs · your-tools/ruplacer
https://github.com/your-tools/ruplacer/actions/workflows/test.yml
Find and replace text in source files. Contribute to your-tools/ruplacer development by creating an account on GitHub.
- 386💥 Blazing fast terminal file manager written in Rust, based on async I/O.
https://github.com/sxyazi/yazi
💥 Blazing fast terminal file manager written in Rust, based on async I/O. - sxyazi/yazi
- 387ASCII terminal hexagonal map roguelike written in Rust
https://github.com/dpc/rhex
ASCII terminal hexagonal map roguelike written in Rust - dpc/rhex
- 388Skytable is a modern scalable NoSQL database with BlueQL, designed for performance, scalability and flexibility. Skytable gives you spaces, models, data types, complex collections and more to build powerful experiences
https://github.com/skytable/skytable
Skytable is a modern scalable NoSQL database with BlueQL, designed for performance, scalability and flexibility. Skytable gives you spaces, models, data types, complex collections and more to build...
- 389A simple timetracker for the command line. It saves a log of all tracked activities as a plaintext file and allows you to create flexible reports.
https://github.com/nikolassv/bartib
A simple timetracker for the command line. It saves a log of all tracked activities as a plaintext file and allows you to create flexible reports. - nikolassv/bartib
- 390Cairo is the first Turing-complete language for creating provable programs for general computation.
https://github.com/starkware-libs/cairo
Cairo is the first Turing-complete language for creating provable programs for general computation. - starkware-libs/cairo
- 391Developer-friendly, serverless vector database for AI applications. Easily add long-term memory to your LLM apps!
https://github.com/lancedb/lancedb
Developer-friendly, serverless vector database for AI applications. Easily add long-term memory to your LLM apps! - lancedb/lancedb
- 392Solidity-Compiler Version Manager
https://github.com/alloy-rs/svm-rs
Solidity-Compiler Version Manager. Contribute to alloy-rs/svm-rs development by creating an account on GitHub.
- 393Warp is a modern, Rust-based terminal with AI built in so you and your team can build great software, faster.
https://github.com/warpdotdev/Warp
Warp is a modern, Rust-based terminal with AI built in so you and your team can build great software, faster. - warpdotdev/Warp
- 394minimalistic command launcher in rust
https://github.com/buster/rrun
minimalistic command launcher in rust. Contribute to buster/rrun development by creating an account on GitHub.
- 395Ethereum Virtual Machine written in rust that is fast and simple to use
https://github.com/bluealloy/revm
Ethereum Virtual Machine written in rust that is fast and simple to use - bluealloy/revm
- 396Lightning-fast and Powerful Code Editor written in Rust
https://github.com/lapce/lapce
Lightning-fast and Powerful Code Editor written in Rust - lapce/lapce
- 397Workflow runs · BinChengZhao/delicate
https://github.com/BinChengZhao/delicate/actions
A lightweight and distributed task scheduling platform written in rust. (一个轻量的分布式的任务调度平台通过rust编写) - Workflow runs · BinChengZhao/delicate
- 398High-level emulator for iPhone OS apps. This repo is used for issues, releases and CI. Submit patches at: https://review.gerrithub.io/admin/repos/touchHLE/touchHLE
https://github.com/touchHLE/touchHLE
High-level emulator for iPhone OS apps. This repo is used for issues, releases and CI. Submit patches at: https://review.gerrithub.io/admin/repos/touchHLE/touchHLE - touchHLE/touchHLE
- 399High performance and high-precision multithreaded StatsD server
https://github.com/avito-tech/bioyino
High performance and high-precision multithreaded StatsD server - avito-tech/bioyino
- 400Backup tool for PC game saves
https://github.com/mtkennerly/ludusavi
Backup tool for PC game saves. Contribute to mtkennerly/ludusavi development by creating an account on GitHub.
- 401Find and replace text in source files
https://github.com/your-tools/ruplacer
Find and replace text in source files. Contribute to your-tools/ruplacer development by creating an account on GitHub.
- 402A secure embedded operating system for microcontrollers
https://github.com/tock/tock
A secure embedded operating system for microcontrollers - tock/tock
- 403A Flash Player emulator written in Rust
https://github.com/ruffle-rs/ruffle
A Flash Player emulator written in Rust. Contribute to ruffle-rs/ruffle development by creating an account on GitHub.
- 404Code at the speed of thought – Zed is a high-performance, multiplayer code editor from the creators of Atom and Tree-sitter.
https://github.com/zed-industries/zed
Code at the speed of thought – Zed is a high-performance, multiplayer code editor from the creators of Atom and Tree-sitter. - zed-industries/zed
- 405Reference client for NEAR Protocol
https://github.com/near/nearcore
Reference client for NEAR Protocol. Contribute to near/nearcore development by creating an account on GitHub.
- 406Workflow runs · qarmin/czkawka
https://github.com/qarmin/czkawka/actions
Multi functional app to find duplicates, empty folders, similar images etc. - Workflow runs · qarmin/czkawka
- 407Visual Studio Code - Code Editing. Redefined
https://code.visualstudio.com/
Visual Studio Code is a code editor redefined and optimized for building and debugging modern web and cloud applications. Visual Studio Code is free and available on your favorite platform - Linux, macOS, and Windows.
- 408Visual Studio Code - Code Editing. Redefined
https://code.visualstudio.com/.
Visual Studio Code is a code editor redefined and optimized for building and debugging modern web and cloud applications. Visual Studio Code is free and available on your favorite platform - Linux, macOS, and Windows.
- 409The Community for Open Collaboration and Innovation | The Eclipse Foundation
https://www.eclipse.org/
The Eclipse Foundation provides our global community of individuals and organisations with a mature, scalable, and business-friendly environment for open source …
- 410404
https://www.rust-lang.org/tools.
A language empowering everyone to build reliable and efficient software.
- 411Even Better TOML - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=tamasfe.even-better-toml
Extension for Visual Studio Code - Fully-featured TOML support
- 412Dependi - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=fill-labs.dependi
Extension for Visual Studio Code - Empowers developers to efficiently manage dependencies and address vulnerabilities in Rust, Go, JavaScript, Typescript, PHP and Python projects.
- 413Sublime Text - the sophisticated text editor for code, markup and prose
https://www.sublimetext.com/
Available on Mac, Windows and Linux
- 414Cross-Shell Prompt
https://starship.rs/
Starship is the minimal, blazing fast, and extremely customizable prompt for any shell! Shows the information you need, while staying sleek and minimal. Quick installation available for Bash, Fish, ZSH, Ion, Tcsh, Elvish, Nu, Xonsh, Cmd, and Powershell.
- 415Shun Sakai / abcrypt · GitLab
https://gitlab.com/sorairolake/abcrypt
The upstream is https://github.com/sorairolake/abcrypt
- 416Prettier · Opinionated Code Formatter
https://prettier.io/
Opinionated Code Formatter
- 417The OPAQUE Asymmetric PAKE Protocol
https://datatracker.ietf.org/doc/draft-krawczyk-cfrg-opaque/
This draft describes the OPAQUE protocol, a secure asymmetric password authenticated key exchange (aPAKE) that supports mutual authentication in a client-server setting without reliance on PKI and with security against pre-computation attacks upon server compromise. Prior aPAKE protocols did not use salt and if they did, the salt was transmitted in the clear from server to user allowing for the building of targeted pre-computed dictionaries. OPAQUE security has been proven by Jarecki et al. (Eurocrypt 2018) in a strong and universally composable formal model of aPAKE security. In addition, the protocol provides forward secrecy and the ability to hide the password from the server even during password registration. Strong security, versatility through modularity, good performance, and an array of additional features make OPAQUE a natural candidate for practical use and for adoption as a standard. To this end, this draft presents several instantiations of OPAQUE and ways of integrating OPAQUE with TLS. This draft presents a high-level description of OPAQUE, highlighting its components and modular design. It also provides the basis for a specification for standardization but a detailed specification ready for implementation is beyond the scope of this document. Implementers of OPAQUE should ONLY follow the precise specification in the upcoming draft-irtf-cfrg-opaque.
- 418CodeLLDB - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=vadimcn.vscode-lldb
Extension for Visual Studio Code - A native debugger powered by LLDB. Debug C++, Rust and other compiled languages.
- 419Cyril Plisko / pager-rs · GitLab
https://gitlab.com/imp/pager-rs
Helps pipe your output through an external pager
- 420esp-rs
https://github.com/esp-rs
Libraries, crates and examples for using Rust on Espressif SoC's - esp-rs
- 421Prettier - Code formatter (Rust) - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=jinxdash.prettier-rust
Extension for Visual Studio Code - Prettier Rust is a code formatter that autocorrects bad syntax
- 422IntelliJ IDEA – the Leading Java and Kotlin IDE
https://www.jetbrains.com/idea/
IntelliJ IDEA is undoubtedly the top-choice IDE for software developers. It makes Java and Kotlin development a more productive and enjoyable experience.
- 423OpenCL - The Open Standard for Parallel Programming of Heterogeneous Systems
https://www.khronos.org/opencl/
OpenCL™ (Open Computing Language) is an open, royalty-free standard for cross-platform, parallel programming of diverse accelerators found in supercomputers, cloud servers, personal computers, mobile devices and embedded platforms. OpenCL greatly improves the speed and responsiveness of a wide spectrum of applications in numerous market categories including professional creative tools,
- 424Shun Sakai / scryptenc-rs · GitLab
https://gitlab.com/sorairolake/scryptenc-rs
The upstream is https://github.com/sorairolake/scryptenc-rs
- 425CI · Workflow runs · frewsxcv/cargo-all-features
https://github.com/frewsxcv/cargo-all-features/actions/workflows/ci.yml
A Cargo subcommand to build and test all feature flag combinations. - CI · Workflow runs · frewsxcv/cargo-all-features
- 426Very small rust docker image
https://github.com/kpcyrd/mini-docker-rust
Very small rust docker image. Contribute to kpcyrd/mini-docker-rust development by creating an account on GitHub.
- 427Workflow runs · matthiaskrgr/cargo-cache
https://github.com/matthiaskrgr/cargo-cache/actions
manage cargo cache (${CARGO_HOME}, ~/.cargo/), print sizes of dirs and remove dirs selectively - Workflow runs · matthiaskrgr/cargo-cache
- 428Pluggable and configurable code formatting platform written in Rust.
https://github.com/dprint/dprint
Pluggable and configurable code formatting platform written in Rust. - dprint/dprint
- 429A cross platform minimalistic text user interface
https://github.com/ivanceras/titik
A cross platform minimalistic text user interface. Contribute to ivanceras/titik development by creating an account on GitHub.
- 430Cross-platform, low level networking using the Rust programming language.
https://github.com/libpnet/libpnet
Cross-platform, low level networking using the Rust programming language. - libpnet/libpnet
- 431Dataframe structure and operations in Rust
https://github.com/kernelmachine/utah
Dataframe structure and operations in Rust. Contribute to kernelmachine/utah development by creating an account on GitHub.
- 432A fast, offline, reverse geocoder
https://github.com/gx0r/rrgeo
A fast, offline, reverse geocoder. Contribute to gx0r/rrgeo development by creating an account on GitHub.
- 433enum-map
https://codeberg.org/xfix/enum-map
A map with C-like enum keys represented internally as an array
- 434contain-rs
https://github.com/contain-rs
Extension of Rust's std::collections. contain-rs has 24 repositories available. Follow their code on GitHub.
- 435Rust library for interacting with the UDisks2 DBus API
https://github.com/pop-os/dbus-udisks2
Rust library for interacting with the UDisks2 DBus API - pop-os/dbus-udisks2
- 436Qonfucius / Aragog · GitLab
https://gitlab.com/qonfucius/aragog
A simple lightweight ArangoDB ODM and OGM for Rust. https://aragog.rs
- 437An implementation of the IETF QUIC protocol
https://github.com/aws/s2n-quic
An implementation of the IETF QUIC protocol. Contribute to aws/s2n-quic development by creating an account on GitHub.
- 438Plotly for Rust
https://github.com/plotly/plotly.rs
Plotly for Rust. Contribute to plotly/plotly.rs development by creating an account on GitHub.
- 439NNTP client for Rust
https://github.com/mattnenterprise/rust-nntp
NNTP client for Rust. Contribute to mattnenterprise/rust-nntp development by creating an account on GitHub.
- 440Extra iterator adaptors, iterator methods, free functions, and macros.
https://github.com/rust-itertools/itertools
Extra iterator adaptors, iterator methods, free functions, and macros. - rust-itertools/itertools
- 441Distributed stream processing engine in Rust
https://github.com/ArroyoSystems/arroyo
Distributed stream processing engine in Rust. Contribute to ArroyoSystems/arroyo development by creating an account on GitHub.
- 442🧰 The Rust SQL Toolkit. An async, pure Rust SQL crate featuring compile-time checked queries without a DSL. Supports PostgreSQL, MySQL, and SQLite.
https://github.com/launchbadge/sqlx
🧰 The Rust SQL Toolkit. An async, pure Rust SQL crate featuring compile-time checked queries without a DSL. Supports PostgreSQL, MySQL, and SQLite. - launchbadge/sqlx
- 443A pure rust YAML implementation.
https://github.com/chyh1990/yaml-rust
A pure rust YAML implementation. Contribute to chyh1990/yaml-rust development by creating an account on GitHub.
- 444CI · Workflow runs · emilk/egui
https://github.com/emilk/egui/actions?workflow=CI
egui: an easy-to-use immediate mode GUI in Rust that runs on both web and native - CI · Workflow runs · emilk/egui
- 445CI · Workflow runs · iddm/toornament-rs
https://github.com/iddm/toornament-rs/actions/workflows/ci.yml
A rust library for toornament.com service. Contribute to iddm/toornament-rs development by creating an account on GitHub.
- 446TDS 7.2+ (Microsoft SQL Server) driver for Rust
https://github.com/prisma/tiberius
TDS 7.2+ (Microsoft SQL Server) driver for Rust. Contribute to prisma/tiberius development by creating an account on GitHub.
- 447Workflow runs · tnballo/scapegoat
https://github.com/tnballo/scapegoat/actions
Safe, fallible, embedded-friendly ordered set/map via a scapegoat tree. Validated against BTreeSet/BTreeMap. - Workflow runs · tnballo/scapegoat
- 448piston/LICENSE at master · PistonDevelopers/piston
https://github.com/PistonDevelopers/piston/blob/master/LICENSE
A modular game engine written in Rust. Contribute to PistonDevelopers/piston development by creating an account on GitHub.
- 449A no_std + serde compatible message library for Rust
https://github.com/jamesmunns/postcard
A no_std + serde compatible message library for Rust - jamesmunns/postcard
- 450Zero-copy deserialization framework for Rust
https://github.com/rkyv/rkyv
Zero-copy deserialization framework for Rust. Contribute to rkyv/rkyv development by creating an account on GitHub.
- 451CouchDB client-side library for the Rust programming language
https://github.com/chill-rs/chill
CouchDB client-side library for the Rust programming language - chill-rs/chill
- 452Workflow runs · redox-os/orbtk
https://github.com/redox-os/orbtk/actions
The Rust UI-Toolkit. Contribute to redox-os/orbtk development by creating an account on GitHub.
- 453FlatBuffers compiler (flatc) as API (with focus on transparent `.fbs` to `.rs` code-generation via Cargo build scripts integration)
https://github.com/frol/flatc-rust
FlatBuffers compiler (flatc) as API (with focus on transparent `.fbs` to `.rs` code-generation via Cargo build scripts integration) - frol/flatc-rust
- 454Specs - Parallel ECS
https://github.com/amethyst/specs
Specs - Parallel ECS. Contribute to amethyst/specs development by creating an account on GitHub.
- 455Email test server for development, written in Rust
https://github.com/tweedegolf/mailcrab
Email test server for development, written in Rust - tweedegolf/mailcrab
- 456A small charting/visualization tool and partial vega implementation for Rust
https://github.com/saresend/Gust
A small charting/visualization tool and partial vega implementation for Rust - saresend/Gust
- 457Alpaca - Developer-first API for Stock, Options, Crypto Trading
https://alpaca.markets/
Alpaca's easy to use APIs allow developers and businesses to trade algorithms, build apps and embed investing into their services.
- 458Rust · Workflow runs · redis-rs/redis-rs
https://github.com/redis-rs/redis-rs/actions/workflows/rust.yml
Redis library for rust. Contribute to redis-rs/redis-rs development by creating an account on GitHub.
- 459Async-friendly QUIC implementation in Rust
https://github.com/quinn-rs/quinn
Async-friendly QUIC implementation in Rust. Contribute to quinn-rs/quinn development by creating an account on GitHub.
- 460Are we game yet?
https://arewegameyet.rs
A guide to the Rust game development ecosystem.
- 461Rust library to parse mail files
https://github.com/staktrace/mailparse
Rust library to parse mail files. Contribute to staktrace/mailparse development by creating an account on GitHub.
- 462Rusty Object Notation
https://github.com/ron-rs/ron
Rusty Object Notation. Contribute to ron-rs/ron development by creating an account on GitHub.
- 463A refreshingly simple data-driven game engine built in Rust
https://github.com/bevyengine/bevy
A refreshingly simple data-driven game engine built in Rust - bevyengine/bevy
- 464An XML library in Rust
https://github.com/netvl/xml-rs
An XML library in Rust. Contribute to netvl/xml-rs development by creating an account on GitHub.
- 465Yet Another Serializer/Deserializer
https://github.com/media-io/yaserde
Yet Another Serializer/Deserializer. Contribute to media-io/yaserde development by creating an account on GitHub.
- 466An auxiliary serde library providing helpful functions for serialisation and deserialisation for containers, struct fields and others.
https://github.com/iddm/serde-aux/
An auxiliary serde library providing helpful functions for serialisation and deserialisation for containers, struct fields and others. - iddm/serde-aux
- 467A Rust ASN.1 (DER) serializer.
https://github.com/alex/rust-asn1
A Rust ASN.1 (DER) serializer. Contribute to alex/rust-asn1 development by creating an account on GitHub.
- 468Dataframes powered by a multithreaded, vectorized query engine, written in Rust
https://github.com/pola-rs/polars
Dataframes powered by a multithreaded, vectorized query engine, written in Rust - pola-rs/polars
- 469Rust persistent data structures
https://github.com/orium/rpds
Rust persistent data structures. Contribute to orium/rpds development by creating an account on GitHub.
- 470Rust · Workflow runs · amethyst/bracket-lib
https://github.com/amethyst/bracket-lib/actions/workflows/rust.yml
The Roguelike Toolkit (RLTK), implemented for Rust. - Rust · Workflow runs · amethyst/bracket-lib
- 471An etcd client library for Rust.
https://github.com/jimmycuadra/rust-etcd
An etcd client library for Rust. Contribute to jimmycuadra/rust-etcd development by creating an account on GitHub.
- 472Workflow runs · mjovanc/njord
https://github.com/mjovanc/njord/actions/workflows/ci.yml
A lightweight ORM library for Rust ⛵. Contribute to mjovanc/njord development by creating an account on GitHub.
- 473test library · Workflow runs · tauri-apps/tauri
https://github.com/tauri-apps/tauri/actions?query=workflow%3A%22test+library%22
Build smaller, faster, and more secure desktop applications with a web frontend. - test library · Workflow runs · tauri-apps/tauri
- 474Strongly typed JSON library for Rust
https://github.com/serde-rs/json
Strongly typed JSON library for Rust. Contribute to serde-rs/json development by creating an account on GitHub.
- 475RocksDB | A persistent key-value store
https://rocksdb.org/
RocksDB is an embeddable persistent key-value store for fast storage.
- 476Two dimensional grid data structure
https://github.com/becheran/grid
Two dimensional grid data structure. Contribute to becheran/grid development by creating an account on GitHub.
- 477Hypergraph is data structure library to create a directed hypergraph in which a hyperedge can join any number of vertices.
https://github.com/yamafaktory/hypergraph
Hypergraph is data structure library to create a directed hypergraph in which a hyperedge can join any number of vertices. - yamafaktory/hypergraph
- 478Asyncronous Rust Mysql driver based on Tokio.
https://github.com/blackbeam/mysql_async
Asyncronous Rust Mysql driver based on Tokio. Contribute to blackbeam/mysql_async development by creating an account on GitHub.
- 479Workflow runs · softprops/dynomite
https://github.com/softprops/dynomite/actions
⚡🦀 🧨 make your rust types fit DynamoDB and visa versa - Workflow runs · softprops/dynomite
- 480Minimal and opinionated eBPF tooling for the Rust ecosystem
https://github.com/libbpf/libbpf-rs
Minimal and opinionated eBPF tooling for the Rust ecosystem - libbpf/libbpf-rs
- 481A CSV parser for Rust, with Serde support.
https://github.com/BurntSushi/rust-csv
A CSV parser for Rust, with Serde support. Contribute to BurntSushi/rust-csv development by creating an account on GitHub.
- 482Antimony Topology Builder library
https://github.com/antimonyproject/antimony
Antimony Topology Builder library. Contribute to antimonyproject/antimony development by creating an account on GitHub.
- 483⚡🦀 🧨 make your rust types fit DynamoDB and visa versa
https://github.com/softprops/dynomite
⚡🦀 🧨 make your rust types fit DynamoDB and visa versa - softprops/dynomite
- 484Pipelines · Friz64 / erupt · GitLab
https://gitlab.com/Friz64/erupt/-/pipelines
Vulkan API bindings
- 485Generic array types in Rust
https://github.com/fizyk20/generic-array
Generic array types in Rust. Contribute to fizyk20/generic-array development by creating an account on GitHub.
- 486High-performance runtime for data analytics applications
https://github.com/weld-project/weld
High-performance runtime for data analytics applications - weld-project/weld
- 487The gRPC library for Rust built on C Core library and futures
https://github.com/tikv/grpc-rs
The gRPC library for Rust built on C Core library and futures - tikv/grpc-rs
- 488build · Workflow runs · Mnwa/ms
https://github.com/Mnwa/ms/actions?query=workflow%3Abuild
Fast abstraction for converting human-like times into milliseconds. - build · Workflow runs · Mnwa/ms
- 489Pure Rust library for Apache ZooKeeper built on tokio
https://github.com/krojew/rust-zookeeper
Pure Rust library for Apache ZooKeeper built on tokio - krojew/rust-zookeeper
- 490🐝 terminal mail client, mirror of https://git.meli-email.org/meli/meli.git https://crates.io/crates/meli
https://github.com/meli/meli
🐝 terminal mail client, mirror of https://git.meli-email.org/meli/meli.git https://crates.io/crates/meli - meli/meli
- 491A highly scalable MySQL Proxy framework written in Rust
https://github.com/AgilData/mysql-proxy-rs
A highly scalable MySQL Proxy framework written in Rust - AgilData/mysql-proxy-rs
- 492Strongly typed YAML library for Rust
https://github.com/dtolnay/serde-yaml
Strongly typed YAML library for Rust. Contribute to dtolnay/serde-yaml development by creating an account on GitHub.
- 493🥧 Savoury implementation of the QUIC transport protocol and HTTP/3
https://github.com/cloudflare/quiche
🥧 Savoury implementation of the QUIC transport protocol and HTTP/3 - cloudflare/quiche
- 494The official MongoDB Rust Driver
https://github.com/mongodb/mongo-rust-driver
The official MongoDB Rust Driver. Contribute to mongodb/mongo-rust-driver development by creating an account on GitHub.
- 495Commits · master · Qonfucius / Aragog · GitLab
https://gitlab.com/qonfucius/aragog/-/commits/master
A simple lightweight ArangoDB ODM and OGM for Rust. https://aragog.rs
- 496Blazingly fast file search library built in Rust
https://github.com/ParthJadhav/Rust_Search
Blazingly fast file search library built in Rust. Contribute to ParthJadhav/Rust_Search development by creating an account on GitHub.
- 497Serialization framework for Rust
https://github.com/serde-rs/serde
Serialization framework for Rust. Contribute to serde-rs/serde development by creating an account on GitHub.
- 498Cargo Build & Test · Workflow runs · DJDuque/pgfplots
https://github.com/DJDuque/pgfplots/actions/workflows/rust.yml
PGFPlots code generator. Contribute to DJDuque/pgfplots development by creating an account on GitHub.
- 499rust wrapper for rocksdb
https://github.com/rust-rocksdb/rust-rocksdb
rust wrapper for rocksdb. Contribute to rust-rocksdb/rust-rocksdb development by creating an account on GitHub.
- 500PGFPlots code generator
https://github.com/djduque/pgfplots
PGFPlots code generator. Contribute to DJDuque/pgfplots development by creating an account on GitHub.
- 501Rust library for reading/writing numbers in big-endian and little-endian.
https://github.com/BurntSushi/byteorder
Rust library for reading/writing numbers in big-endian and little-endian. - BurntSushi/byteorder
- 502Workflow runs · infinyon/fluvio
https://github.com/infinyon/fluvio/actions
Lean and mean distributed stream processing system written in rust and web assembly. Alternative to Kafka + Flink in one. - Workflow runs · infinyon/fluvio
- 503🧭 GraphQL framework for SeaORM
https://github.com/SeaQL/seaography
🧭 GraphQL framework for SeaORM. Contribute to SeaQL/seaography development by creating an account on GitHub.
- 504Workflow runs · tokio-rs/prost
https://github.com/tokio-rs/prost/actions
PROST! a Protocol Buffers implementation for the Rust Language - Workflow runs · tokio-rs/prost
- 505Shun Sakai / nt-time · GitLab
https://gitlab.com/sorairolake/nt-time
The upstream is https://github.com/sorairolake/nt-time
- 506raylib
https://www.raylib.com/
raylib is a simple and easy-to-use library to enjoy videogames programming.
- 507High level FFI binding around the sys mount & umount2 calls, for Rust
https://github.com/pop-os/sys-mount
High level FFI binding around the sys mount & umount2 calls, for Rust - pop-os/sys-mount
- 508Extended attribute library for rust.
https://github.com/Stebalien/xattr
Extended attribute library for rust. Contribute to Stebalien/xattr development by creating an account on GitHub.
- 509Nathan Kent / nng-rs · GitLab
https://gitlab.com/neachdainn/nng-rs
Rust bindings for nanomsg-next-generation
- 510The GTK Project - A free and open-source cross-platform widget toolkit
https://www.gtk.org/
GTK is a free and open-source cross-platform widget toolkit for creating graphical user interfaces.
- 511Rust client for NATS, the cloud native messaging system.
https://github.com/nats-io/nats.rs
Rust client for NATS, the cloud native messaging system. - nats-io/nats.rs
- 512PostgreSQL
https://www.postgresql.org/
The world's most advanced open source database.
- 513PoloDB is an embedded document database.
https://github.com/PoloDB/PoloDB
PoloDB is an embedded document database. Contribute to PoloDB/PoloDB development by creating an account on GitHub.
- 514SurrealDB | The ultimate multi-model database for tomorrow's applications
https://surrealdb.com/
SurrealDB is the ultimate database for tomorrow's serverless, jamstack, single-page, and traditional applications.
- 515Godot Engine - Free and open source 2D and 3D game engine
https://godotengine.org/
Godot provides a huge set of common tools, so you can just focus on making your game without reinventing the wheel.
- 516Rust zeromq bindings.
https://github.com/erickt/rust-zmq
Rust zeromq bindings. Contribute to erickt/rust-zmq development by creating an account on GitHub.
- 517jcreekmore/pem-rs
https://github.com/jcreekmore/pem-rs
Contribute to jcreekmore/pem-rs development by creating an account on GitHub.
- 518An async Redis client for Rust.
https://github.com/aembke/fred.rs
An async Redis client for Rust. Contribute to aembke/fred.rs development by creating an account on GitHub.
- 519MessagePack implementation for Rust / msgpack.org[Rust]
https://github.com/3Hren/msgpack-rust
MessagePack implementation for Rust / msgpack.org[Rust] - 3Hren/msgpack-rust
- 520An SMTP server for test and development environments written in Rust
https://github.com/mailtutan/mailtutan
An SMTP server for test and development environments written in Rust - mailtutan/mailtutan
- 521A crate for interacting with the Alpaca API at alpaca.markets.
https://github.com/d-e-s-o/apca
A crate for interacting with the Alpaca API at alpaca.markets. - d-e-s-o/apca
- 522Friz64 / erupt · GitLab
https://gitlab.com/Friz64/erupt
Vulkan API bindings
- 523Rust-Qt
https://github.com/rust-qt
Qt bindings for Rust language. Rust-Qt has 6 repositories available. Follow their code on GitHub.
- 524Rust color scales library
https://github.com/mazznoer/colorgrad-rs
Rust color scales library. Contribute to mazznoer/colorgrad-rs development by creating an account on GitHub.
- 525A rust drawing library for high quality data plotting for both WASM and native, statically and realtimely 🦀 📈🚀
https://github.com/plotters-rs/plotters
A rust drawing library for high quality data plotting for both WASM and native, statically and realtimely 🦀 📈🚀 - plotters-rs/plotters
- 526Pure Rust library for Apache ZooKeeper built on MIO
https://github.com/bonifaido/rust-zookeeper
Pure Rust library for Apache ZooKeeper built on MIO - bonifaido/rust-zookeeper
- 527A STOMP client in Rust. Compatible with RabbitMQ, ActiveMQ.
https://github.com/zslayton/stomp-rs
A STOMP client in Rust. Compatible with RabbitMQ, ActiveMQ. - zslayton/stomp-rs
- 528Apache DataFusion SQL Query Engine
https://github.com/apache/datafusion
Apache DataFusion SQL Query Engine. Contribute to apache/datafusion development by creating an account on GitHub.
- 529A safe, extensible ORM and Query Builder for Rust
https://github.com/diesel-rs/diesel
A safe, extensible ORM and Query Builder for Rust. Contribute to diesel-rs/diesel development by creating an account on GitHub.
- 530Redis library for rust
https://github.com/redis-rs/redis-rs
Redis library for rust. Contribute to redis-rs/redis-rs development by creating an account on GitHub.
- 531Iggy is the persistent message streaming platform written in Rust, supporting QUIC, TCP and HTTP transport protocols, capable of processing millions of messages per second.
https://github.com/iggy-rs/iggy
Iggy is the persistent message streaming platform written in Rust, supporting QUIC, TCP and HTTP transport protocols, capable of processing millions of messages per second. - iggy-rs/iggy
- 532Temporary file library for rust
https://github.com/Stebalien/tempfile
Temporary file library for rust. Contribute to Stebalien/tempfile development by creating an account on GitHub.
- 533Redis - The Real-time Data Platform
https://redis.io/
Developers love Redis. Unlock the full potential of the Redis database with Redis Enterprise and start building blazing fast apps.
- 534Rust port of simdjson
https://github.com/simd-lite/simd-json
Rust port of simdjson. Contribute to simd-lite/simd-json development by creating an account on GitHub.
- 535▦⧉⊞□ A curated list of links to miniquad/macroquad-related code & resources
https://github.com/ozkriff/awesome-quads
▦⧉⊞□ A curated list of links to miniquad/macroquad-related code & resources - ozkriff/awesome-quads
- 536skade/leveldb
https://github.com/skade/leveldb
Contribute to skade/leveldb development by creating an account on GitHub.
- 537Pipelines · Nathan Kent / nng-rs · GitLab
https://gitlab.com/neachdainn/nng-rs/-/pipelines
Rust bindings for nanomsg-next-generation
- 538Fyrox - A feature-rich game engine built in Rust
https://fyrox.rs/
Fyrox is a feature-rich, production-ready, open-source game engine written in Rust.
- 539Flutter - Build apps for any screen
https://flutter.dev/
Flutter transforms the entire app development process. Build, test, and deploy beautiful mobile, web, desktop, and embedded apps from a single codebase.
- 540Interactive graph visualization widget for rust powered by egui and petgraph
https://github.com/blitzarx1/egui_graphs
Interactive graph visualization widget for rust powered by egui and petgraph - blitzarx1/egui_graphs
- 541ggez/LICENSE at master · ggez/ggez
https://github.com/ggez/ggez/blob/master/LICENSE
Rust library to create a Good Game Easily. Contribute to ggez/ggez development by creating an account on GitHub.
- 542A HTTP Archive format (HAR) serialization & deserialization library, written in Rust.
https://github.com/mandrean/har-rs
A HTTP Archive format (HAR) serialization & deserialization library, written in Rust. - mandrean/har-rs
- 543A binary encoder / decoder implementation in Rust.
https://github.com/bincode-org/bincode
A binary encoder / decoder implementation in Rust. - bincode-org/bincode
- 544A generic connection pool for Rust
https://github.com/sfackler/r2d2
A generic connection pool for Rust. Contribute to sfackler/r2d2 development by creating an account on GitHub.
- 545ndarray: an N-dimensional array with array views, multidimensional slicing, and efficient operations
https://github.com/rust-ndarray/ndarray
ndarray: an N-dimensional array with array views, multidimensional slicing, and efficient operations - rust-ndarray/ndarray
- 546A priority queue for Rust with efficient change function.
https://github.com/garro95/priority-queue
A priority queue for Rust with efficient change function. - GitHub - garro95/priority-queue: A priority queue for Rust with efficient change function.
- 547E-mail delivery library for Rust with DKIM support
https://github.com/stalwartlabs/mail-send
E-mail delivery library for Rust with DKIM support - stalwartlabs/mail-send
- 548Array helpers for Rust's Vector and String types
https://github.com/danielpclark/array_tool
Array helpers for Rust's Vector and String types. Contribute to danielpclark/array_tool development by creating an account on GitHub.
- 549An impish, cross-platform binary parsing crate, written in Rust
https://github.com/m4b/goblin
An impish, cross-platform binary parsing crate, written in Rust - m4b/goblin
- 550Generic extensions for tapping values in Rust.
https://github.com/myrrlyn/tap
Generic extensions for tapping values in Rust. Contribute to myrrlyn/tap development by creating an account on GitHub.
- 551Language Integrated Query in Rust.
https://github.com/StardustDL/Linq-in-Rust
Language Integrated Query in Rust. Contribute to StardustDL/Linq-in-Rust development by creating an account on GitHub.
- 552Rust plotting library using Python (Matplotlib)
https://github.com/cpmech/plotpy
Rust plotting library using Python (Matplotlib). Contribute to cpmech/plotpy development by creating an account on GitHub.
- 553An Embedded NoSQL, Transactional Database Engine
https://github.com/symisc/unqlite
An Embedded NoSQL, Transactional Database Engine. Contribute to symisc/unqlite development by creating an account on GitHub.
- 554Type-safe database access for Rust
https://github.com/Brendonovich/prisma-client-rust
Type-safe database access for Rust. Contribute to Brendonovich/prisma-client-rust development by creating an account on GitHub.
- 555Self-contained distributed software platform for building stateful, massively real-time streaming applications in Rust.
https://github.com/swimos/swim-rust
Self-contained distributed software platform for building stateful, massively real-time streaming applications in Rust. - swimos/swim-rust
- 556tests · Workflow runs · SeaQL/sea-query
https://github.com/SeaQL/sea-query/actions/workflows/rust.yml
🔱 A dynamic SQL query builder for MySQL, Postgres and SQLite - tests · Workflow runs · SeaQL/sea-query
- 557Visualize streams of multimodal data. Fast, easy to use, and simple to integrate. Built in Rust using egui.
https://github.com/rerun-io/rerun
Visualize streams of multimodal data. Fast, easy to use, and simple to integrate. Built in Rust using egui. - rerun-io/rerun
- 558Workflow runs · plotters-rs/plotters
https://github.com/plotters-rs/plotters/actions
A rust drawing library for high quality data plotting for both WASM and native, statically and realtimely 🦀 📈🚀 - Workflow runs · plotters-rs/plotters
- 559CI · Workflow runs · StardustDL/Linq-in-Rust
https://github.com/StardustDL/Linq-in-Rust/actions?query=workflow%3ACI
Language Integrated Query in Rust. Contribute to StardustDL/Linq-in-Rust development by creating an account on GitHub.
- 560PickleDB-rs is a lightweight and simple key-value store. It is a Rust version for Python's PickleDB
https://github.com/seladb/pickledb-rs
PickleDB-rs is a lightweight and simple key-value store. It is a Rust version for Python's PickleDB - seladb/pickledb-rs
- 561Rust high performance xml reader and writer
https://github.com/tafia/quick-xml
Rust high performance xml reader and writer. Contribute to tafia/quick-xml development by creating an account on GitHub.
- 562Challonge REST API Client
https://github.com/iddm/challonge-rs
Challonge REST API Client. Contribute to iddm/challonge-rs development by creating an account on GitHub.
- 563Data plotting library for Rust
https://github.com/milliams/plotlib
Data plotting library for Rust. Contribute to milliams/plotlib development by creating an account on GitHub.
- 564Like Rust's std::path::Path, but UTF-8.
https://github.com/camino-rs/camino
Like Rust's std::path::Path, but UTF-8. Contribute to camino-rs/camino development by creating an account on GitHub.
- 565GeoRust
https://github.com/georust
An ecosystem of geospatial tools and libraries written in Rust - GeoRust
- 566Workflow runs · BinChengZhao/delay-timer
https://github.com/BinChengZhao/delay-timer/actions
Time-manager of delayed tasks. Like crontab, but synchronous asynchronous tasks are possible scheduling, and dynamic add/cancel/remove is supported. - Workflow runs · BinChengZhao/delay-timer
- 567Artem Starikov / tbot · GitLab
https://gitlab.com/SnejUgal/tbot
Make cool Telegram bots with Rust easily. https://tbot.rs
- 568An alternative, typed and simple wavefront format parser and writer.
https://github.com/replicadse/wavefront_rs
An alternative, typed and simple wavefront format parser and writer. - replicadse/wavefront_rs
- 569Rust on Exercism
https://exercism.org/tracks/rust
Get fluent in Rust by solving 98 exercises. And then level up with mentoring from our world-class team.
- 570Rust bindings for iptables
https://github.com/yaa110/rust-iptables
Rust bindings for iptables. Contribute to yaa110/rust-iptables development by creating an account on GitHub.
- 571Pipelines · nyx-space / nyx · GitLab
https://gitlab.com/nyx-space/nyx/-/pipelines
Nyx: Blazing fast high-fidelity astrodynamics for Monte Carlo analyzes of constellations, interplanetary missions, and deep space flight navigation -- https://nyxspace.com/
- 572A Rust library for zero-allocation parsing of binary data.
https://github.com/nrc/zero
A Rust library for zero-allocation parsing of binary data. - nrc/zero
- 573Workflow runs · Keats/tera
https://github.com/Keats/tera/actions
A template engine for Rust based on Jinja2/Django. Contribute to Keats/tera development by creating an account on GitHub.
- 574MetaCall: The ultimate polyglot programming experience.
https://github.com/metacall/core
MetaCall: The ultimate polyglot programming experience. - metacall/core
- 575Workflow runs · microsoft/windows-rs
https://github.com/microsoft/windows-rs/actions
Rust for Windows. Contribute to microsoft/windows-rs development by creating an account on GitHub.
- 576A simple Cross-platform thread schedule and priority library for rust.
https://github.com/iddm/thread-priority/
A simple Cross-platform thread schedule and priority library for rust. - iddm/thread-priority
- 577Workflow runs · retep998/winapi-rs
https://github.com/retep998/winapi-rs/actions/workflows/rust.yml
Rust bindings to Windows API. Contribute to retep998/winapi-rs development by creating an account on GitHub.
- 578paradedb/pg_search at dev · paradedb/paradedb
https://github.com/paradedb/paradedb/tree/dev/pg_search
Postgres for Search and Analytics. Contribute to paradedb/paradedb development by creating an account on GitHub.
- 579CI · Workflow runs · iddm/thread-priority
https://github.com/iddm/thread-priority/actions/workflows/ci.yml
A simple Cross-platform thread schedule and priority library for rust. - CI · Workflow runs · iddm/thread-priority
- 580三体编程语言 Three Body Language written in Rust
https://github.com/rustq/3body-lang
三体编程语言 Three Body Language written in Rust. Contribute to rustq/3body-lang development by creating an account on GitHub.
- 581A community curated list of Rust Language streamers
https://github.com/jamesmunns/awesome-rust-streaming
A community curated list of Rust Language streamers - jamesmunns/awesome-rust-streaming
- 582Workflow runs · meilisearch/meilisearch
https://github.com/meilisearch/MeiliSearch/actions
A lightning-fast search API that fits effortlessly into your apps, websites, and workflow - Workflow runs · meilisearch/meilisearch
- 583Split a string into shell words, like Python's shlex.
https://github.com/comex/rust-shlex
Split a string into shell words, like Python's shlex. - comex/rust-shlex
- 584Rust implementation of a FreeBSD jail library
https://github.com/fubarnetes/libjail-rs/
Rust implementation of a FreeBSD jail library. Contribute to fubarnetes/libjail-rs development by creating an account on GitHub.
- 585Workflow runs · becheran/wildmatch
https://github.com/becheran/wildmatch/actions
Simple string matching with single- and multiple-wildcard operator - Workflow runs · becheran/wildmatch
- 586ci · Workflow runs · fancy-regex/fancy-regex
https://github.com/fancy-regex/fancy-regex/actions/workflows/ci.yml
Rust library for regular expressions using "fancy" features like look-around and backreferences - ci · Workflow runs · fancy-regex/fancy-regex
- 587A GraphQL server library implemented in Rust
https://github.com/async-graphql/async-graphql
A GraphQL server library implemented in Rust. Contribute to async-graphql/async-graphql development by creating an account on GitHub.
- 588Workflow runs · salvo-rs/salvo
https://github.com/salvo-rs/salvo/actions
A powerful web framework built with a simplified design. - Workflow runs · salvo-rs/salvo
- 589Rust · Workflow runs · hannobraun/inotify-rs
https://github.com/hannobraun/inotify-rs/actions/workflows/rust.yml
Idiomatic inotify wrapper for the Rust programming language - Rust · Workflow runs · hannobraun/inotify-rs
- 590Actix Web is a powerful, pragmatic, and extremely fast web framework for Rust.
https://github.com/actix/actix-web
Actix Web is a powerful, pragmatic, and extremely fast web framework for Rust. - actix/actix-web
- 591(Read-only) Generate n-grams
https://github.com/pwoolcoc/ngrams
(Read-only) Generate n-grams. Contribute to pwoolcoc/ngrams development by creating an account on GitHub.
- 592Rust bindings to Windows API
https://github.com/retep998/winapi-rs
Rust bindings to Windows API. Contribute to retep998/winapi-rs development by creating an account on GitHub.
- 593Rhai - An embedded scripting language for Rust.
https://github.com/rhaiscript/rhai
Rhai - An embedded scripting language for Rust. Contribute to rhaiscript/rhai development by creating an account on GitHub.
- 594Rust friendly bindings to *nix APIs
https://github.com/nix-rust/nix
Rust friendly bindings to *nix APIs. Contribute to nix-rust/nix development by creating an account on GitHub.
- 595RustConf 2017 - Shipping a Solid Rust Crate by Michael Gattozzi
https://www.youtube.com/watch?v=t4CyEKb-ywA
Shipping a Solid Rust Crate by Michael Gattozzi There's a lot more to releasing a quality crate than just the code. Automating the testing to make sure nothi...
- 596The WebSocket Protocol
https://datatracker.ietf.org/doc/rfc6455/
The WebSocket Protocol enables two-way communication between a client running untrusted code in a controlled environment to a remote host that has opted-in to communications from that code. The security model used for this is the origin-based security model commonly used by web browsers. The protocol consists of an opening handshake followed by basic message framing, layered over TCP. The goal of this technology is to provide a mechanism for browser-based applications that need two-way communication with servers that does not rely on opening multiple HTTP connections (e.g., using XMLHttpRequest or <iframe>s and long polling). [STANDARDS-TRACK]
- 597Safe Rust bindings to POSIX-ish APIs
https://github.com/bytecodealliance/rustix
Safe Rust bindings to POSIX-ish APIs. Contribute to bytecodealliance/rustix development by creating an account on GitHub.
- 598Simple, extendable and embeddable scripting language.
https://github.com/sagiegurari/duckscript
Simple, extendable and embeddable scripting language. - sagiegurari/duckscript
- 599Lisp dialect scripting and extension language for Rust programs
https://github.com/murarth/ketos
Lisp dialect scripting and extension language for Rust programs - murarth/ketos
- 600Rust in Action
https://www.manning.com/books/rust-in-action
Rust in Action</i> introduces the Rust programming language by exploring numerous systems programming concepts and techniques. You'll be learning Rust by delving into how computers work under the hood. You'll find yourself playing with persistent storage, memory, networking and even tinkering with CPU instructions. The book takes you through using Rust to extend other applications and teaches you tricks to write blindingly fast code. You'll also discover parallel and concurrent programming. Filled to the brim with real-life use cases and scenarios, you'll go beyond the Rust syntax and see what Rust has to offer in real-world use cases.
- 601Workflow runs · ducaale/xh
https://github.com/ducaale/xh/actions
Friendly and fast tool for sending HTTP requests. Contribute to ducaale/xh development by creating an account on GitHub.
- 602Build fast web applications with Rust.
https://github.com/leptos-rs/leptos
Build fast web applications with Rust. Contribute to leptos-rs/leptos development by creating an account on GitHub.
- 603A cross-platform serial port library in Rust. Provides a blocking I/O interface and port enumeration including USB device information.
https://github.com/serialport/serialport-rs
A cross-platform serial port library in Rust. Provides a blocking I/O interface and port enumeration including USB device information. - serialport/serialport-rs
- 604Workflow runs · nathanbabcock/ffmpeg-sidecar
https://github.com/nathanbabcock/ffmpeg-sidecar/actions/workflows/rust.yml
Wrap a standalone FFmpeg binary in an intuitive Iterator interface. 🏍 - Workflow runs · nathanbabcock/ffmpeg-sidecar
- 605tests · Workflow runs · ardaku/whoami
https://github.com/ardaku/whoami/actions/workflows/ci.yml
Rust crate to get the current user and environment. - tests · Workflow runs · ardaku/whoami
- 606The reference implementation of the Linux FUSE (Filesystem in Userspace) interface
https://github.com/libfuse/libfuse
The reference implementation of the Linux FUSE (Filesystem in Userspace) interface - libfuse/libfuse
- 607Perlin: An Efficient and Ergonomic Document Search-Engine
https://github.com/CurrySoftware/perlin
Perlin: An Efficient and Ergonomic Document Search-Engine - CurrySoftware/perlin
- 608Rust API to the OS X Hypervisor framework for hardware-accelerated virtualization
https://github.com/saurvs/hypervisor-rs
Rust API to the OS X Hypervisor framework for hardware-accelerated virtualization - saurvs/hypervisor-rs
- 609Workflow runs · graphql-rust/graphql-client
https://github.com/graphql-rust/graphql-client/actions
Typed, correct GraphQL requests and responses in Rust - Workflow runs · graphql-rust/graphql-client
- 610Rust in Motion
https://www.manning.com/livevideo/rust-in-motion?a_aid=cnichols&a_bid=6a993c2e
See it. Do it. Learn it! In Rust in Motion</i>, premier Rust experts Carol Nichols and Jake Goulding, introduce you to the Rust programming language! Designed for modern systems programming, Rust delivers impressive speed and thread-safe concurrency. As coauthor of The Rust Programming Language</i>, Carol literally helped write “The Book,” as the Rust community affectionately calls it. Jake created the Rust FFI Omnibus</i>, and he’s also the #1 contributor to the Rust tag on Stack Overflow. If you’re ready to get started writing production-quality lightning-fast systems code, this liveVideo course is for you!
- 611😋 Make your own blog!
https://github.com/leven-the-blog/leven
😋 Make your own blog! Contribute to leven-the-blog/leven development by creating an account on GitHub.
- 612The Computer Language Benchmarks Game: Rust implementations
https://github.com/TeXitoi/benchmarksgame-rs
The Computer Language Benchmarks Game: Rust implementations - TeXitoi/benchmarksgame-rs
- 613CI · Workflow runs · iddm/urlshortener-rs
https://github.com/iddm/urlshortener-rs/actions/workflows/ci.yml
A very-very simple url shortener (client) for Rust. - CI · Workflow runs · iddm/urlshortener-rs
- 614Dyer is designed for reliable, flexible and fast web crawling, providing some high-level, comprehensive features without compromising speed.
https://github.com/hominee/dyer
Dyer is designed for reliable, flexible and fast web crawling, providing some high-level, comprehensive features without compromising speed. - hominee/dyer
- 615Rust library for regular expressions using "fancy" features like look-around and backreferences
https://github.com/fancy-regex/fancy-regex
Rust library for regular expressions using "fancy" features like look-around and backreferences - fancy-regex/fancy-regex
- 616Computer Hardware Crash Course - So You Want to Build a Language VM
https://blog.subnetzero.io/post/building-language-vm-part-00/
Covers general elements of computer hardware useful to know before reading the rest of the tutorials
- 617:pencil: Compile-time HTML templates for Rust
https://github.com/lambda-fairy/maud
:pencil: Compile-time HTML templates for Rust. Contribute to lambda-fairy/maud development by creating an account on GitHub.
- 618Represent large sets and maps compactly with finite state transducers.
https://github.com/BurntSushi/fst
Represent large sets and maps compactly with finite state transducers. - BurntSushi/fst
- 619Advanced Rust quantum computer simulator
https://github.com/beneills/quantum
Advanced Rust quantum computer simulator. Contribute to beneills/quantum development by creating an account on GitHub.
- 620Fast suffix arrays for Rust (with Unicode support).
https://github.com/BurntSushi/suffix
Fast suffix arrays for Rust (with Unicode support). - BurntSushi/suffix
- 621Rust Compiled Templates with static-file handling
https://github.com/kaj/ructe
Rust Compiled Templates with static-file handling. Contribute to kaj/ructe development by creating an account on GitHub.
- 622A fast and secure runtime for WebAssembly
https://github.com/bytecodealliance/wasmtime
A fast and secure runtime for WebAssembly. Contribute to bytecodealliance/wasmtime development by creating an account on GitHub.
- 623Time-manager of delayed tasks. Like crontab, but synchronous asynchronous tasks are possible scheduling, and dynamic add/cancel/remove is supported.
https://github.com/BinChengZhao/delay-timer
Time-manager of delayed tasks. Like crontab, but synchronous asynchronous tasks are possible scheduling, and dynamic add/cancel/remove is supported. - BinChengZhao/delay-timer
- 624✈️ a private, authenticated, permissioned cargo registry
https://github.com/w4/chartered
✈️ a private, authenticated, permissioned cargo registry - w4/chartered
- 625MetaCall / core · GitLab
https://gitlab.com/metacall/core
A library for providing inter-language foreign function interface calls.
- 626Paul Woolcock / soup · GitLab
https://gitlab.com/pwoolcoc/soup
Like BeautifulSoup, but for rust
- 627Hands-on Rust
https://pragprog.com/titles/hwrust/hands-on-rust/
Make fun games as you learn the Rust programming language through a series of hands-on game development tutorials and real-world use of core language skills.
- 628The GameLisp scripting language
https://github.com/fleabitdev/glsp
The GameLisp scripting language. Contribute to fleabitdev/glsp development by creating an account on GitHub.
- 629A fast monadic-style parser combinator designed to work on stable Rust.
https://github.com/m4rw3r/chomp
A fast monadic-style parser combinator designed to work on stable Rust. - m4rw3r/chomp
- 630VMM userspace for illumos bhyve
https://github.com/oxidecomputer/propolis
VMM userspace for illumos bhyve. Contribute to oxidecomputer/propolis development by creating an account on GitHub.
- 631Workflow runs · Daniel-Liu-c0deb0t/triple_accel
https://github.com/Daniel-Liu-c0deb0t/triple_accel/actions
Rust edit distance routines accelerated using SIMD. Supports fast Hamming, Levenshtein, restricted Damerau-Levenshtein, etc. distance calculations and string search. - Workflow runs · Daniel-Liu-c0...
- 632Over 550 flashcards to learn Rust from first principles. Written in markdown with script to convert them to an Anki deck or PDF file.
https://github.com/ad-si/Rust-Flashcards
Over 550 flashcards to learn Rust from first principles. Written in markdown with script to convert them to an Anki deck or PDF file. - ad-si/Rust-Flashcards
- 633Private Cargo Registry
https://cloudsmith.com/product/formats/cargo-registry
Cloudsmith is your solution for private, secure Cargo registries with cloud-native performance, accessible using native tools. Start your free trial today.
- 634Cross-platform library to fetch system information
https://github.com/GuillaumeGomez/sysinfo
Cross-platform library to fetch system information - GuillaumeGomez/sysinfo
- 635Workflow runs · rhaiscript/rhai
https://github.com/rhaiscript/rhai/actions
Rhai - An embedded scripting language for Rust. Contribute to rhaiscript/rhai development by creating an account on GitHub.
- 636A Rust framework for creating web apps
https://github.com/seed-rs/seed
A Rust framework for creating web apps. Contribute to seed-rs/seed development by creating an account on GitHub.
- 637CI macOS · Workflow runs · FedericoBruzzone/tdlib-rs
https://github.com/FedericoBruzzone/tdlib-rs/actions/workflows/ci-macos.yml
Rust wrapper around the Telegram Database Library 🦀 - CI macOS · Workflow runs · FedericoBruzzone/tdlib-rs
- 638Text calculator with support for units and conversion
https://github.com/probablykasper/cpc
Text calculator with support for units and conversion - probablykasper/cpc
- 639A versatile web framework and library for building client-side and server-side web applications
https://github.com/ivanceras/sauron
A versatile web framework and library for building client-side and server-side web applications - ivanceras/sauron
- 640A lightning-fast search API that fits effortlessly into your apps, websites, and workflow
https://github.com/meilisearch/MeiliSearch
A lightning-fast search API that fits effortlessly into your apps, websites, and workflow - meilisearch/meilisearch
- 641The Elegant Parser
https://github.com/pest-parser/pest
The Elegant Parser. Contribute to pest-parser/pest development by creating an account on GitHub.
- 642Multilingual implementation of RAKE algorithm for Rust
https://github.com/yaa110/rake-rs
Multilingual implementation of RAKE algorithm for Rust - yaa110/rake-rs
- 643A macro-based html builder for rust
https://github.com/Stebalien/horrorshow-rs
A macro-based html builder for rust. Contribute to Stebalien/horrorshow-rs development by creating an account on GitHub.
- 644CI Linux · Workflow runs · FedericoBruzzone/tdlib-rs
https://github.com/FedericoBruzzone/tdlib-rs/actions/workflows/ci-linux.yml
Rust wrapper around the Telegram Database Library 🦀 - CI Linux · Workflow runs · FedericoBruzzone/tdlib-rs
- 645An HTTP library for Rust
https://github.com/hyperium/hyper
An HTTP library for Rust. Contribute to hyperium/hyper development by creating an account on GitHub.
- 646A super-easy, composable, web server framework for warp speeds.
https://github.com/seanmonstar/warp
A super-easy, composable, web server framework for warp speeds. - seanmonstar/warp
- 647openapi schema serialization for rust
https://github.com/softprops/openapi
openapi schema serialization for rust. Contribute to softprops/openapi development by creating an account on GitHub.
- 648A simple and lightweight fuzzy search engine that works in memory, searching for similar strings (a pun here).
https://github.com/andylokandy/simsearch-rs
A simple and lightweight fuzzy search engine that works in memory, searching for similar strings (a pun here). - andylokandy/simsearch-rs
- 649A template engine for Rust based on Jinja2/Django
https://github.com/Keats/tera
A template engine for Rust based on Jinja2/Django. Contribute to Keats/tera development by creating an account on GitHub.
- 650Libfprint library for Rust
https://github.com/alvaroparker/libfprint-rs
Libfprint library for Rust. Contribute to AlvaroParker/libfprint-rs development by creating an account on GitHub.
- 651Workflow runs · replicadse/wavefront_rs
https://github.com/replicadse/wavefront_rs/actions
An alternative, typed and simple wavefront format parser and writer. - Workflow runs · replicadse/wavefront_rs
- 652Learn Rust - Best Rust Tutorials | Hackr.io
https://hackr.io/tutorials/learn-rust
Learning Rust? Check out these best online Rust courses and tutorials recommended by the programming community. Pick the tutorial as per your learning style: video tutorials or a book. Free course or paid. Tutorials for beginners or advanced learners. Check Rust community's reviews & comments.
- 653A lib crate for gathering system info such as cpu, distro, environment, kernel, etc in Rust.
https://github.com/Phate6660/nixinfo
A lib crate for gathering system info such as cpu, distro, environment, kernel, etc in Rust. - Phate6660/nixinfo
- 654CI · Workflow runs · GuillaumeGomez/sysinfo
https://github.com/GuillaumeGomez/sysinfo/actions/workflows/CI.yml
Cross-platform library to fetch system information - CI · Workflow runs · GuillaumeGomez/sysinfo
- 655Refactoring to Rust
https://www.manning.com/books/refactoring-to-rust
Get the speed and reliability of Rust libraries, functions, and high-performance features through incremental adoption without rewriting your codebase from scratch.</b> In Refactoring to Rust</i> you will learn to: Create Rust libraries you can call from other programming languages</li> Integrate Rust functions with code in other languages</li> Use Rust’s ownership and borrowing system to write high performance code</li> Handle errors as values using Rust’s enums</li> Minimize unnecessary memory usage with Rust’s multiple string types</li> Boost performance with Rust concurrency and async event processing</li> Create Rust HTTP services</li> </ul> Refactoring to Rust</i> teaches you how to take advantage of Rust’s easy-to-use interoperating mechanisms. Learn practical code-mixing techniques like embedding Rust libraries into apps written in other languages. This practical guide emphasises techniques for incrementally refactoring performance-critical code to Rust while keeping the rest of your application in its original language.
- 656Rust templating with Handlebars
https://github.com/sunng87/handlebars-rust
Rust templating with Handlebars. Contribute to sunng87/handlebars-rust development by creating an account on GitHub.
- 657CI · Workflow runs · w4/chartered
https://github.com/w4/chartered/actions/workflows/ci.yml
✈️ a private, authenticated, permissioned cargo registry - CI · Workflow runs · w4/chartered
- 658A Rust library for generically joining iterables with a separator
https://github.com/Lucretiel/joinery
A Rust library for generically joining iterables with a separator - Lucretiel/joinery
- 659Rust parser combinator framework
https://github.com/rust-bakery/nom
Rust parser combinator framework. Contribute to rust-bakery/nom development by creating an account on GitHub.
- 660Douman / yukikaze · GitLab
https://gitlab.com/Douman/yukikaze
Beautiful and elegant Yukikaze is little HTTP client library based on hyper
- 661Rust · Workflow runs · comex/rust-shlex
https://github.com/comex/rust-shlex/actions/workflows/test.yml
Split a string into shell words, like Python's shlex. - Rust · Workflow runs · comex/rust-shlex
- 662Type-safe, compiled Jinja-like templates for Rust
https://github.com/djc/askama
Type-safe, compiled Jinja-like templates for Rust. Contribute to djc/askama development by creating an account on GitHub.
- 663Simple string matching with single- and multiple-wildcard operator
https://github.com/becheran/wildmatch
Simple string matching with single- and multiple-wildcard operator - becheran/wildmatch
- 664Unit tests · Workflow runs · quickwit-oss/tantivy
https://github.com/quickwit-oss/tantivy/actions/workflows/test.yml
Tantivy is a full-text search engine library inspired by Apache Lucene and written in Rust - Unit tests · Workflow runs · quickwit-oss/tantivy
- 665Commits · master · Artem Starikov / tbot · GitLab
https://gitlab.com/SnejUgal/tbot/-/commits/master
Make cool Telegram bots with Rust easily. https://tbot.rs
- 666Compiler front-end foundation technology.
https://github.com/Eliah-Lakhin/lady-deirdre
Compiler front-end foundation technology. Contribute to Eliah-Lakhin/lady-deirdre development by creating an account on GitHub.
- 667Yarte stands for Yet Another Rusty Template Engine
https://github.com/zzau13/yarte
Yarte stands for Yet Another Rusty Template Engine - zzau13/yarte
- 668carols10cents - Overview
https://github.com/carols10cents
carols10cents has 165 repositories available. Follow their code on GitHub.
- 669Rust wrapper around the Telegram Database Library 🦀
https://github.com/FedericoBruzzone/tdlib-rs
Rust wrapper around the Telegram Database Library 🦀 - FedericoBruzzone/tdlib-rs
- 670A native Rust port of Google's robots.txt parser and matcher C++ library.
https://github.com/Folyd/robotstxt
A native Rust port of Google's robots.txt parser and matcher C++ library. - Folyd/robotstxt
- 671Source code for the Mun language and runtime.
https://github.com/mun-lang/mun
Source code for the Mun language and runtime. Contribute to mun-lang/mun development by creating an account on GitHub.
- 672Wrap a standalone FFmpeg binary in an intuitive Iterator interface. 🏍
https://github.com/nathanbabcock/ffmpeg-sidecar
Wrap a standalone FFmpeg binary in an intuitive Iterator interface. 🏍 - nathanbabcock/ffmpeg-sidecar
- 673Rust Servers, Services, and Apps
https://www.manning.com/books/rust-servers-services-and-apps
Deliver fast, reliable, and maintainable applications by building backend servers, services, and frontends all in nothing but Rust.</b><br/><br/> In Rust Servers, Services, and Apps</i>, you’ll learn:<br/><br/> Developing database-backed web services in Rust</li> Building and securing RESTful APIs</li> Writing server-side web applications in Rust</li> Measuring and benchmarking web service performance</li> Packaging and deploying web services</li> Full-stack Rust applications</li> </ul> The blazingly fast, safe, and efficient Rust language has been voted “most loved” for multiple consecutive years on the StackOverflow survey. Rust Server, Services, and Apps</i> shows you why! Inside, you’ll build web servers, RESTful services, server-rendered apps, and client frontends just using Rust. You’ll learn to write code with small and predictable resource footprints, and build high-performing applications with unmatched safety and reliability.
- 674Different benchmarks of different implementations measured mainly to evaluate the performance of the wtx project
https://github.com/c410-f3r/wtx-bench
Different benchmarks of different implementations measured mainly to evaluate the performance of the wtx project - c410-f3r/wtx-bench
- 675Shun Sakai / sysexits-rs · GitLab
https://gitlab.com/sorairolake/sysexits-rs
The upstream is https://github.com/sorairolake/sysexits-rs
- 676KCL Programming Language (CNCF Sandbox Project). https://kcl-lang.io
https://github.com/kcl-lang/kcl
KCL Programming Language (CNCF Sandbox Project). https://kcl-lang.io - kcl-lang/kcl
- 677Rust edit distance routines accelerated using SIMD. Supports fast Hamming, Levenshtein, restricted Damerau-Levenshtein, etc. distance calculations and string search.
https://github.com/Daniel-Liu-c0deb0t/triple_accel
Rust edit distance routines accelerated using SIMD. Supports fast Hamming, Levenshtein, restricted Damerau-Levenshtein, etc. distance calculations and string search. - Daniel-Liu-c0deb0t/triple_accel
- 678CI · Workflow runs · null8626/decancer
https://github.com/null8626/decancer/actions/workflows/CI.yml
A library that removes common unicode confusables/homoglyphs from strings. - CI · Workflow runs · null8626/decancer
- 679Natural language detection library for Rust. Try demo online: https://whatlang.org/
https://github.com/greyblake/whatlang-rs
Natural language detection library for Rust. Try demo online: https://whatlang.org/ - greyblake/whatlang-rs
- 680Shiva library: Implementation in Rust of a parser and generator for documents of any type
https://github.com/igumnoff/shiva
Shiva library: Implementation in Rust of a parser and generator for documents of any type - igumnoff/shiva
- 681A typed parser generator embedded in Rust code for Parsing Expression Grammars
https://github.com/ptal/oak
A typed parser generator embedded in Rust code for Parsing Expression Grammars - ptal/oak
- 682Elastic tabstops for Rust.
https://github.com/BurntSushi/tabwriter
Elastic tabstops for Rust. Contribute to BurntSushi/tabwriter development by creating an account on GitHub.
- 683Rust for Windows
https://github.com/microsoft/windows-rs
Rust for Windows. Contribute to microsoft/windows-rs development by creating an account on GitHub.
- 684A parser combinator library for Rust
https://github.com/Marwes/combine
A parser combinator library for Rust. Contribute to Marwes/combine development by creating an account on GitHub.
- 685Rust crate to get the current user and environment.
https://github.com/ardaku/whoami
Rust crate to get the current user and environment. - ardaku/whoami
- 686Take your first steps with Rust - Training
https://learn.microsoft.com/en-us/training/paths/rust-first-steps/
Interested in learning a new programming language that's growing in use and popularity? Start here! Lay the foundation of knowledge you need to build fast and effective programs in Rust.
- 687An embeddable dynamic programming language for Rust.
https://github.com/rune-rs/rune
An embeddable dynamic programming language for Rust. - rune-rs/rune
- 688An implementation of regular expressions for Rust. This implementation uses finite automata and guarantees linear time matching on all inputs.
https://github.com/rust-lang/regex
An implementation of regular expressions for Rust. This implementation uses finite automata and guarantees linear time matching on all inputs. - rust-lang/regex
- 689Build software better, together
https://github.com/flosse/rust-web-framework-comparison.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 690CI · Workflow runs · bytecodealliance/wasmtime
https://github.com/bytecodealliance/wasmtime/actions?query=workflow%3ACI
A fast and secure runtime for WebAssembly. Contribute to bytecodealliance/wasmtime development by creating an account on GitHub.
- 691Workflow runs · sagiegurari/duckscript
https://github.com/sagiegurari/duckscript/actions
Simple, extendable and embeddable scripting language. - Workflow runs · sagiegurari/duckscript
- 692A static, type inferred and embeddable language written in Rust.
https://github.com/gluon-lang/gluon
A static, type inferred and embeddable language written in Rust. - gluon-lang/gluon
- 693⏮ ⏯ ⏭ A Rust library for easily navigating forward, backward or randomly through the lines of huge files.
https://github.com/ps1dr3x/easy_reader
⏮ ⏯ ⏭ A Rust library for easily navigating forward, backward or randomly through the lines of huge files. - ps1dr3x/easy_reader
- 694Workflow runs · teloxide/teloxide
https://github.com/teloxide/teloxide/actions
🤖 An elegant Telegram bots framework for Rust. Contribute to teloxide/teloxide development by creating an account on GitHub.
- 695JSON Web Token implementation in Rust.
https://github.com/GildedHonour/frank_jwt
JSON Web Token implementation in Rust. Contribute to GildedHonour/frank_jwt development by creating an account on GitHub.
- 696Blazing fast dead simple static site generator
https://github.com/grego/blades
Blazing fast dead simple static site generator. Contribute to grego/blades development by creating an account on GitHub.
- 697static site generator from markdown files(markdown 格式静态博客生成器)
https://github.com/FuGangqiang/mdblog.rs
static site generator from markdown files(markdown 格式静态博客生成器) - FuGangqiang/mdblog.rs
- 698A Rust library to extract useful data from HTML documents, suitable for web scraping.
https://github.com/utkarshkukreti/select.rs
A Rust library to extract useful data from HTML documents, suitable for web scraping. - utkarshkukreti/select.rs
- 699Workflow runs · rust-scraper/scraper
https://github.com/rust-scraper/scraper/actions
HTML parsing and querying with CSS selectors. Contribute to rust-scraper/scraper development by creating an account on GitHub.
- 700mgattozzi - Overview
https://github.com/mgattozzi
"A bad programmer" according to the Orange Site, he/him - mgattozzi
- 701A simple full-stack web framework for Rust
https://github.com/saru-tora/anansi
A simple full-stack web framework for Rust. Contribute to saru-tora/anansi development by creating an account on GitHub.
- 702🦀 A peer-reviewed collection of articles/talks/repos which teach concise, idiomatic Rust.
https://github.com/mre/idiomatic-rust
🦀 A peer-reviewed collection of articles/talks/repos which teach concise, idiomatic Rust. - mre/idiomatic-rust
- 703Ratchet is a fast, robust, lightweight and fully asynchronous implementation of RFC6455 (The WebSocket protocol).
https://github.com/swimos/ratchet
Ratchet is a fast, robust, lightweight and fully asynchronous implementation of RFC6455 (The WebSocket protocol). - swimos/ratchet
- 704timClicks - Overview
https://github.com/timClicks
On the planet to build a better planet. timClicks has 219 repositories available. Follow their code on GitHub.
- 705A Rust library for the Discord API.
https://github.com/serenity-rs/serenity
A Rust library for the Discord API. Contribute to serenity-rs/serenity development by creating an account on GitHub.
- 706A flexible web framework that promotes stability, safety, security and speed.
https://github.com/gotham-rs/gotham
A flexible web framework that promotes stability, safety, security and speed. - gotham-rs/gotham
- 707The missing batteries of Rust
https://github.com/brson/stdx
The missing batteries of Rust. Contribute to brson/stdx development by creating an account on GitHub.
- 708A catalogue of Rust design patterns, anti-patterns and idioms
https://github.com/rust-unofficial/patterns
A catalogue of Rust design patterns, anti-patterns and idioms - rust-unofficial/patterns
- 709Installer Backend
https://github.com/pop-os/distinst/
Installer Backend. Contribute to pop-os/distinst development by creating an account on GitHub.
- 710Rust Macro which loads files into the rust binary at compile time during release and loads the file from the fs during dev.
https://github.com/pyrossh/rust-embed
Rust Macro which loads files into the rust binary at compile time during release and loads the file from the fs during dev. - pyrossh/rust-embed
- 711A Computer Science Curriculum with Rust flavor!
https://github.com/AbdesamedBendjeddou/Rusty-CS
A Computer Science Curriculum with Rust flavor! Contribute to AbdesamedBendjeddou/Rusty-CS development by creating an account on GitHub.
- 712A powerful web framework built with a simplified design.
https://github.com/salvo-rs/salvo
A powerful web framework built with a simplified design. - salvo-rs/salvo
- 713The Software Pro's Best Kept Secret.
https://app.codecrafters.io/tracks/rust
Real-world proficiency projects designed for experienced engineers. Develop software craftsmanship by recreating popular devtools from scratch.
- 714🤖 An elegant Telegram bots framework for Rust
https://github.com/teloxide/teloxide/
🤖 An elegant Telegram bots framework for Rust. Contribute to teloxide/teloxide development by creating an account on GitHub.
- 715The enterprise-ready webhooks service 🦀
https://github.com/svix/svix-webhooks
The enterprise-ready webhooks service 🦀. Contribute to svix/svix-webhooks development by creating an account on GitHub.
- 716A fast static site generator in a single binary with everything built-in. https://www.getzola.org
https://github.com/getzola/zola
A fast static site generator in a single binary with everything built-in. https://www.getzola.org - getzola/zola
- 717Interactive visualizations of Rust at compile-time and run-time
https://github.com/cognitive-engineering-lab/aquascope
Interactive visualizations of Rust at compile-time and run-time - cognitive-engineering-lab/aquascope
- 718Fully async-await http server framework
https://github.com/richerarc/saphir
Fully async-await http server framework. Contribute to richerarc/saphir development by creating an account on GitHub.
- 719Rust query string parser with nesting support
https://github.com/s-panferov/queryst
Rust query string parser with nesting support. Contribute to s-panferov/queryst development by creating an account on GitHub.
- 720Sōzu HTTP reverse proxy, configurable at runtime, fast and safe, built in Rust. It is awesome!
https://github.com/sozu-proxy/sozu
Sōzu HTTP reverse proxy, configurable at runtime, fast and safe, built in Rust. It is awesome! - sozu-proxy/sozu
- 721Static site generator written in Rust
https://github.com/cobalt-org/cobalt.rs
Static site generator written in Rust. Contribute to cobalt-org/cobalt.rs development by creating an account on GitHub.
- 722Lightweight stream-based WebSocket implementation for Rust.
https://github.com/snapview/tungstenite-rs
Lightweight stream-based WebSocket implementation for Rust. - snapview/tungstenite-rs
- 723A WebSocket (RFC6455) library written in Rust
https://github.com/websockets-rs/rust-websocket
A WebSocket (RFC6455) library written in Rust. Contribute to websockets-rs/rust-websocket development by creating an account on GitHub.
- 724Low level HTTP server library in Rust
https://github.com/tiny-http/tiny-http
Low level HTTP server library in Rust. Contribute to tiny-http/tiny-http development by creating an account on GitHub.
- 725Command-line client for WebSockets, like netcat (or curl) for ws:// with advanced socat-like functions
https://github.com/vi/websocat
Command-line client for WebSockets, like netcat (or curl) for ws:// with advanced socat-like functions - vi/websocat
- 726REST-like API micro-framework for Rust. Works with Iron.
https://github.com/rustless/rustless
REST-like API micro-framework for Rust. Works with Iron. - rustless/rustless
- 727shepmaster - Overview
https://github.com/shepmaster
shepmaster has 261 repositories available. Follow their code on GitHub.
- 728JsonPath engine written in Rust. Webassembly and Javascript support too
https://github.com/freestrings/jsonpath
JsonPath engine written in Rust. Webassembly and Javascript support too - freestrings/jsonpath
- 729CI · Workflow runs · poem-web/poem
https://github.com/poem-web/poem/actions/workflows/ci.yml
A full-featured and easy-to-use web framework with the Rust programming language. - CI · Workflow runs · poem-web/poem
- 730Optimize, speed, scale your microservices and save money 💵
https://github.com/graphul-rs/graphul
Optimize, speed, scale your microservices and save money 💵 - graphul-rs/graphul
- 731Implementing (part of) a BitTorrent client in Rust
https://www.youtube.com/watch?v=jf_ddGnum_4
In this stream, we're doing the "implement BitTorrent" challenge from https://app.codecrafters.io/join?via=jonhoo in Rust. Essentially, we're implementing a ...
- 732Learn Rust by 500 lines code
https://github.com/cuppar/rtd
Learn Rust by 500 lines code. Contribute to cuppar/rtd development by creating an account on GitHub.
- 733Simple, Fast, Code first and Compile time generated OpenAPI documentation for Rust
https://github.com/juhaku/utoipa
Simple, Fast, Code first and Compile time generated OpenAPI documentation for Rust - juhaku/utoipa
- 734A collection of different transport implementations and related tools focused primarily on web technologies.
https://github.com/c410-f3r/wtx
A collection of different transport implementations and related tools focused primarily on web technologies. - c410-f3r/wtx
- 735Friendly and fast tool for sending HTTP requests
https://github.com/ducaale/xh
Friendly and fast tool for sending HTTP requests. Contribute to ducaale/xh development by creating an account on GitHub.
- 736HTML parsing and querying with CSS selectors
https://github.com/rust-scraper/scraper
HTML parsing and querying with CSS selectors. Contribute to rust-scraper/scraper development by creating an account on GitHub.
- 737A fast, boilerplate free, web framework for Rust
https://github.com/carllerche/tower-web
A fast, boilerplate free, web framework for Rust. Contribute to carllerche/tower-web development by creating an account on GitHub.
- 738An Extensible, Concurrent Web Framework for Rust
https://github.com/iron/iron
An Extensible, Concurrent Web Framework for Rust. Contribute to iron/iron development by creating an account on GitHub.
- 739A full-featured and easy-to-use web framework with the Rust programming language.
https://github.com/poem-web/poem
A full-featured and easy-to-use web framework with the Rust programming language. - poem-web/poem
- 740Rust grammar tool libraries and binaries
https://github.com/softdevteam/grmtools/
Rust grammar tool libraries and binaries. Contribute to softdevteam/grmtools development by creating an account on GitHub.
- 741A flexible template engine for Rust
https://github.com/rustache/rustache
A flexible template engine for Rust. Contribute to rustache/rustache development by creating an account on GitHub.
- 742Tantivy is a full-text search engine library inspired by Apache Lucene and written in Rust
https://github.com/quickwit-oss/tantivy
Tantivy is a full-text search engine library inspired by Apache Lucene and written in Rust - quickwit-oss/tantivy
- 743An efficient and powerful Rust library for word wrapping text.
https://github.com/mgeisler/textwrap
An efficient and powerful Rust library for word wrapping text. - mgeisler/textwrap
- 744CI · Workflow runs · tokio-rs/axum
https://github.com/tokio-rs/axum/actions/workflows/CI.yml
Ergonomic and modular web framework built with Tokio, Tower, and Hyper - CI · Workflow runs · tokio-rs/axum
- 745Niko Matsakis "Rust: Hack Without Fear!"
https://www.youtube.com/watch?v=lO1z-7cuRYI
http://cppnow.org—Presentation Slides, PDFs, Source Code and other presenter materials are available at: https://github.com/boostcon/cppnow_presentations_201...
- 746An expressjs inspired web framework for Rust
https://github.com/nickel-org/nickel.rs/
An expressjs inspired web framework for Rust. Contribute to nickel-org/nickel.rs development by creating an account on GitHub.
- 747Leetcode Solutions in Rust, Advent of Code Solutions in Rust and more
https://github.com/warycat/rustgym
Leetcode Solutions in Rust, Advent of Code Solutions in Rust and more - warycat/rustgym
- 748An opinionated framework for creating REST-like APIs in Ruby.
https://github.com/ruby-grape/grape
An opinionated framework for creating REST-like APIs in Ruby. - ruby-grape/grape
- 749Web framework in Rust
https://github.com/tomaka/rouille
Web framework in Rust. Contribute to tomaka/rouille development by creating an account on GitHub.
- 750CI · Workflow runs · bytecodealliance/rustix
https://github.com/bytecodealliance/rustix/actions?query=workflow%3ACI
Safe Rust bindings to POSIX-ish APIs. Contribute to bytecodealliance/rustix development by creating an account on GitHub.
- 751Oso is a batteries-included framework for building authorization in your application.
https://github.com/osohq/oso
Oso is a batteries-included framework for building authorization in your application. - osohq/oso
- 752LR(1) parser generator for Rust
https://github.com/lalrpop/lalrpop
LR(1) parser generator for Rust. Contribute to lalrpop/lalrpop development by creating an account on GitHub.
- 753Common Expression Language interpreter written in Rust
https://github.com/clarkmcc/cel-rust
Common Expression Language interpreter written in Rust - clarkmcc/cel-rust
- 754nikomatsakis - Overview
https://github.com/nikomatsakis
nikomatsakis has 246 repositories available. Follow their code on GitHub.
- 755thebracket - Overview
https://github.com/thebracket/
Author of Hands-on Rust, Rust Brain Teasers, Advanced Hands-on Rust. Rust person at Ardan Labs. Chief Product Officer at LibreQoS. - thebracket
- 756Valitron is a rust validation, support ergonomics, functional and configurable
https://github.com/tu6ge/valitron
Valitron is a rust validation, support ergonomics, functional and configurable - tu6ge/valitron
- 757Load cookies from your web browsers
https://github.com/thewh1teagle/rookie
Load cookies from your web browsers. Contribute to thewh1teagle/rookie development by creating an account on GitHub.
- 758CI Windows · Workflow runs · FedericoBruzzone/tdlib-rs
https://github.com/FedericoBruzzone/tdlib-rs/actions/workflows/ci-windows.yml
Rust wrapper around the Telegram Database Library 🦀 - CI Windows · Workflow runs · FedericoBruzzone/tdlib-rs
- 759Utoipa build · Workflow runs · juhaku/utoipa
https://github.com/juhaku/utoipa/actions/workflows/build.yaml
Simple, Fast, Code first and Compile time generated OpenAPI documentation for Rust - Utoipa build · Workflow runs · juhaku/utoipa
- 760Sincere is a micro web framework for Rust(stable) based on hyper and multithreading
https://github.com/danclive/sincere
Sincere is a micro web framework for Rust(stable) based on hyper and multithreading - danclive/sincere
- 761A very-very simple url shortener (client) for Rust.
https://github.com/iddm/urlshortener-rs
A very-very simple url shortener (client) for Rust. - iddm/urlshortener-rs
- 762Hand curated advice and pointers for getting started with Rust
https://github.com/jondot/rust-how-do-i-start
Hand curated advice and pointers for getting started with Rust - jondot/rust-how-do-i-start
- 763Rust library for filesystems in userspace (FUSE)
https://github.com/zargony/fuse-rs
Rust library for filesystems in userspace (FUSE). Contribute to zargony/fuse-rs development by creating an account on GitHub.
- 764A rusty dynamically typed scripting language
https://github.com/PistonDevelopers/dyon
A rusty dynamically typed scripting language. Contribute to PistonDevelopers/dyon development by creating an account on GitHub.
- 765An easy and powerful Rust HTTP Client
https://github.com/seanmonstar/reqwest
An easy and powerful Rust HTTP Client. Contribute to seanmonstar/reqwest development by creating an account on GitHub.
- 766A lightweight web framework built on hyper, implemented in Rust language.
https://github.com/miketang84/sapper
A lightweight web framework built on hyper, implemented in Rust language. - miketang84/sapper
- 767Typed, correct GraphQL requests and responses in Rust
https://github.com/graphql-rust/graphql-client
Typed, correct GraphQL requests and responses in Rust - graphql-rust/graphql-client
- 768List of Rust books
https://github.com/sger/RustBooks
List of Rust books. Contribute to sger/RustBooks development by creating an account on GitHub.
- 769A Rust web framework
https://github.com/cargonauts-rs/cargonauts
A Rust web framework. Contribute to cargonauts-rs/cargonauts development by creating an account on GitHub.
- 770Lightweight, event-driven WebSockets for Rust.
https://github.com/housleyjk/ws-rs
Lightweight, event-driven WebSockets for Rust. Contribute to housleyjk/ws-rs development by creating an account on GitHub.
- 771A web framework for Rust.
https://github.com/rwf2/Rocket
A web framework for Rust. Contribute to rwf2/Rocket development by creating an account on GitHub.
- 772Interactively Visualizing Ownership and Borrowing for Rust
https://github.com/rustviz/rustviz
Interactively Visualizing Ownership and Borrowing for Rust - rustviz/rustviz
- 773Idiomatic inotify wrapper for the Rust programming language
https://github.com/hannobraun/inotify-rs
Idiomatic inotify wrapper for the Rust programming language - hannobraun/inotify-rs
- 774Development · Workflow runs · osohq/oso
https://github.com/osohq/oso/actions?query=branch%3Amain+workflow%3ADevelopment
Oso is a batteries-included framework for building authorization in your application. - Development · Workflow runs · osohq/oso
- 775CI · Workflow runs · hyperium/hyper
https://github.com/hyperium/hyper/actions?query=workflow%3ACI
An HTTP library for Rust. Contribute to hyperium/hyper development by creating an account on GitHub.
- 776Next-generation framework for composable applications in Rust.
https://github.com/zino-rs/zino
Next-generation framework for composable applications in Rust. - zino-rs/zino
- 777:crab: Small exercises to get you used to reading and writing Rust code!
https://github.com/rust-lang/rustlings
:crab: Small exercises to get you used to reading and writing Rust code! - rust-lang/rustlings
- 778Rust bindings to libcurl
https://github.com/alexcrichton/curl-rust
Rust bindings to libcurl. Contribute to alexcrichton/curl-rust development by creating an account on GitHub.
- 779A bunch of links to blog posts, articles, videos, etc for learning Rust
https://github.com/ctjhoa/rust-learning
A bunch of links to blog posts, articles, videos, etc for learning Rust - ctjhoa/rust-learning
- 780GraphQL server library for Rust
https://github.com/graphql-rust/juniper
GraphQL server library for Rust. Contribute to graphql-rust/juniper development by creating an account on GitHub.
- 781Rust explained using easy English
https://github.com/Dhghomon/easy_rust
Rust explained using easy English. Contribute to Dhghomon/easy_rust development by creating an account on GitHub.
- 782Ergonomic and modular web framework built with Tokio, Tower, and Hyper
https://github.com/tokio-rs/axum
Ergonomic and modular web framework built with Tokio, Tower, and Hyper - tokio-rs/axum
- 783An incremental parsing system for programming tools
https://github.com/tree-sitter/tree-sitter
An incremental parsing system for programming tools - tree-sitter/tree-sitter
- 784CI · Workflow runs · sozu-proxy/sozu
https://github.com/sozu-proxy/sozu/actions/workflows/ci.yml
Sōzu HTTP reverse proxy, configurable at runtime, fast and safe, built in Rust. It is awesome! - CI · Workflow runs · sozu-proxy/sozu
- 785Parsing Expression Grammar (PEG) parser generator for Rust
https://github.com/kevinmehall/rust-peg
Parsing Expression Grammar (PEG) parser generator for Rust - kevinmehall/rust-peg
- 786A library that removes common unicode confusables/homoglyphs from strings.
https://github.com/null8626/decancer
A library that removes common unicode confusables/homoglyphs from strings. - null8626/decancer
Related Articlesto learn about angular.
- 1Why Rust? A Beginner's Guide to Rust Programming Language
- 2Mastering Rust Ownership and Borrowing: Key Concepts
- 3Building Web APIs with Rust and Actix Web: A Beginner’s Guide
- 4Rust with WebAssembly (Wasm): Bringing Rust to the Browser
- 5Writing High-Performance System Utilities with Rust
- 6Memory Safety and Performance: Rust for Low-Level Programming
- 7Concurrency in Rust: Understanding async/await and Multithreading
- 8Optimizing Rust Code for Maximum Performance: Tips and Techniques
- 9Building a Real-Time Chat Application with Rust and Tokio
- 10Developing a Blockchain with Rust: A Beginner's Guide
FAQ'sto learn more about Angular JS.
mail [email protected] to add more queries here 🔍.
- 1
where is rust programming language used
- 2
which companies are using rust programming language
- 3
is rust programming language free
- 4
what is rust programming language used for
- 5
what programming language is rust written in
- 6
how popular is rust programming language
- 7
is rust a good programming language
- 8
is rust programming easy to learn
- 9
who owns rust programming language
- 10
is rust the best programming language
- 11
is rust programming language cross platform
- 12
what is rust in programming
- 13
where is rust used
- 14
how to learn rust programming
- 15
who wrote rust programming language
- 16
what can you do with rust programming language
- 17
did rust console bp wipe
- 18
why rust programming language is used
- 19
what does rust programming language do
- 20
what can rust programming language do
- 21
where is rust programming used
- 22
what is rust programming used for
- 23
can rust replace java
- 24
is rust programming language dying
- 25
how to start rust programming language
- 26
who created rust programming language
- 27
when to use rust language
- 28
why is rust programming language so popular
- 29
what is rust programming good for
- 30
what can i use rust programming language for
- 31
how rust programming language works
- 32
will rust replace c++ reddit
- 33
- 34
how good is rust programming language
- 35
when to use rust programming language
- 36
what is special about rust programming language
- 37
what is rust used for programming
- 38
how does rust programming language work
- 39
what can i do with rust programming language
- 40
why i hate rust programming language
- 41
what is rust programming language good for
- 42
where is rust language used
- 43
does tesla use rust programming language
- 44
what can i build with rust programming language
- 45
why learn rust programming
- 46
how old is rust programming language
- 47
is rust programming worth it
- 48
how to install rust programming language
- 49
can rust replace python
- 50
does rust support object oriented programming
- 51
where is rust used in production
- 52
is rust a programming language
- 53
who uses rust programming language
- 54
when was rust programming language released
- 55
why rust is bad programming language
- 56
is rust programming still popular
- 57
how to use rust programming language
- 58
how to get good at rust programming
- 59
is rust programming hard to learn
- 60
who invented rust programming language
- 61
where to learn rust programming language
- 62
is rust programming in demand
- 63
have rust servers wiped
- 64
will rust replace c#
- 65
which companies use rust programming language
- 66
what type of programming language is rust
- 67
who made rust programming language
- 68
does rust support functional programming
- 69
when did rust programming language come out
- 70
why rust is the best programming language
- 71
can you do functional programming in rust
- 72
is rust programming free
- 73
should i use rust
- 74
did rust console wipe today
- 75
how to make a programming language in rust
- 76
how long does it take to learn rust programming
- 77
what is the rust programming language good for
- 78
why rust programming language
- 79
is rust programming language easy to learn
- 80
what does rust do programming
- 81
will rust kill c++
- 82
when was rust programming language created
- 83
why is rust programming language called rust
More Sitesto check out once you're finished browsing here.
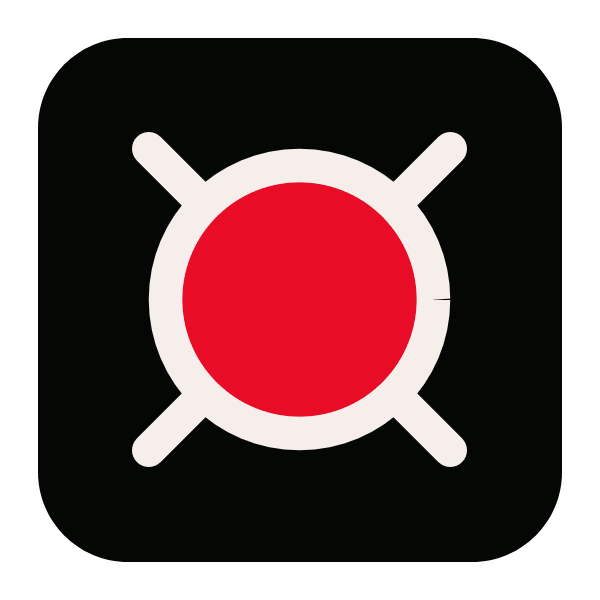
https://www.0x3d.site/
0x3d is designed for aggregating information.
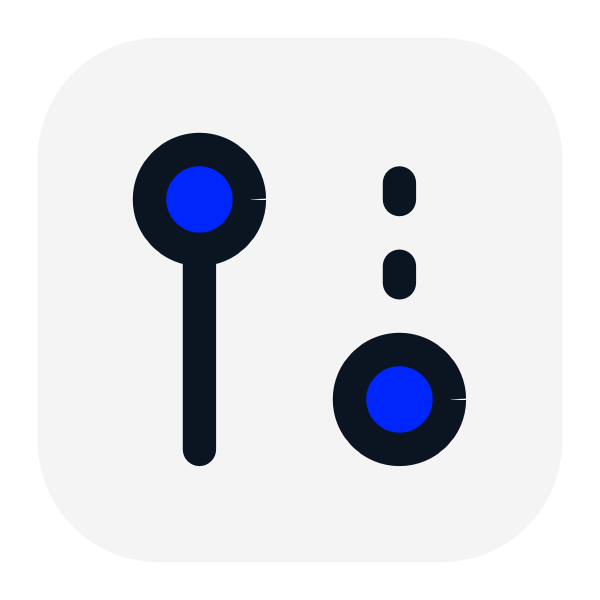
https://nodejs.0x3d.site/
NodeJS Online Directory

https://cross-platform.0x3d.site/
Cross Platform Online Directory
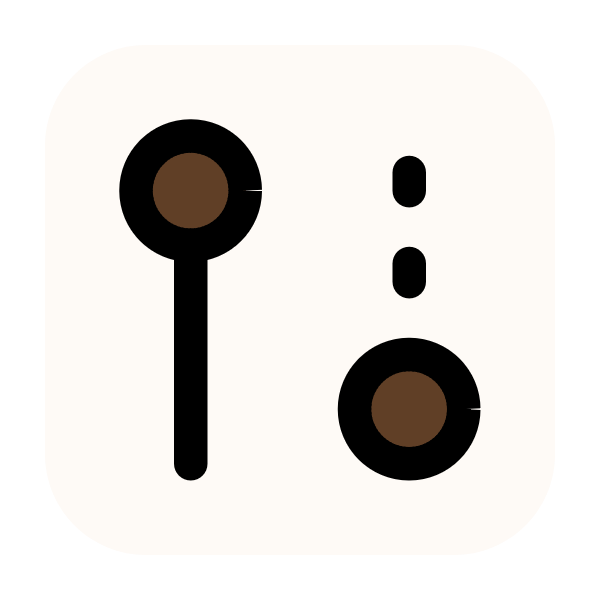
https://open-source.0x3d.site/
Open Source Online Directory
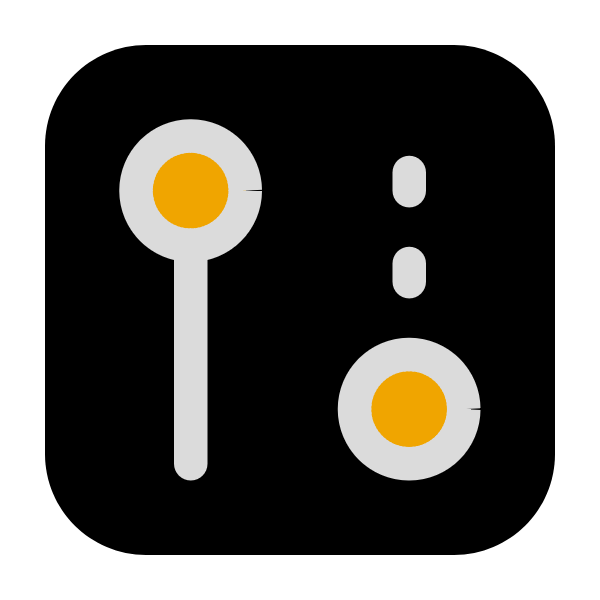
https://analytics.0x3d.site/
Analytics Online Directory
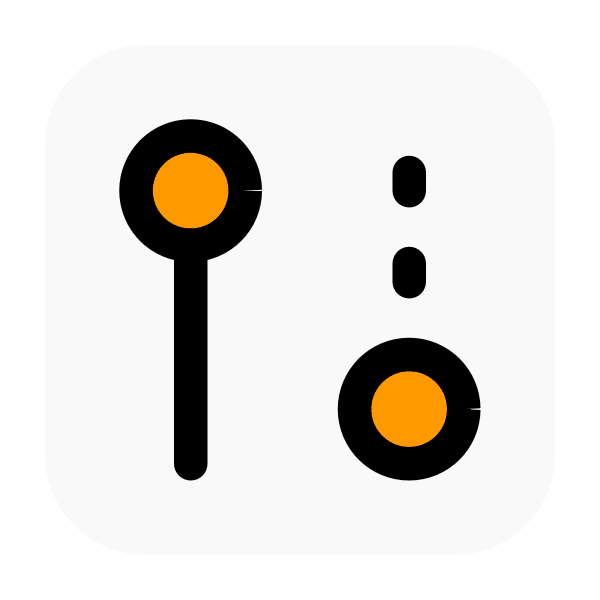
https://javascript.0x3d.site/
JavaScript Online Directory

https://golang.0x3d.site/
GoLang Online Directory
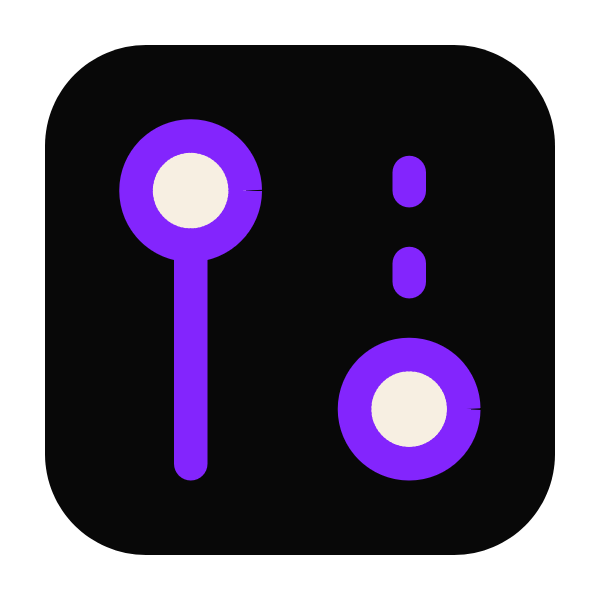
https://python.0x3d.site/
Python Online Directory

https://swift.0x3d.site/
Swift Online Directory

https://rust.0x3d.site/
Rust Online Directory
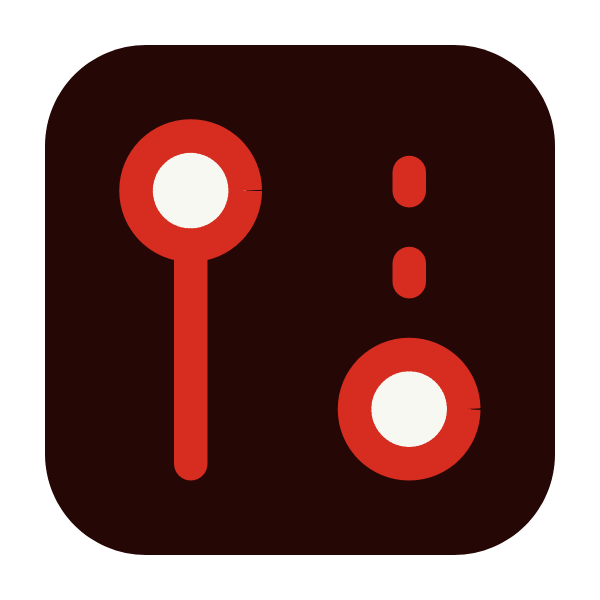
https://scala.0x3d.site/
Scala Online Directory

https://ruby.0x3d.site/
Ruby Online Directory

https://clojure.0x3d.site/
Clojure Online Directory
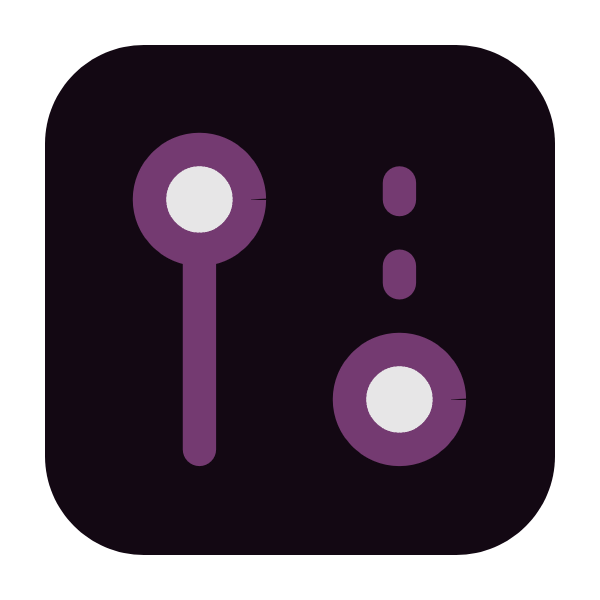
https://elixir.0x3d.site/
Elixir Online Directory
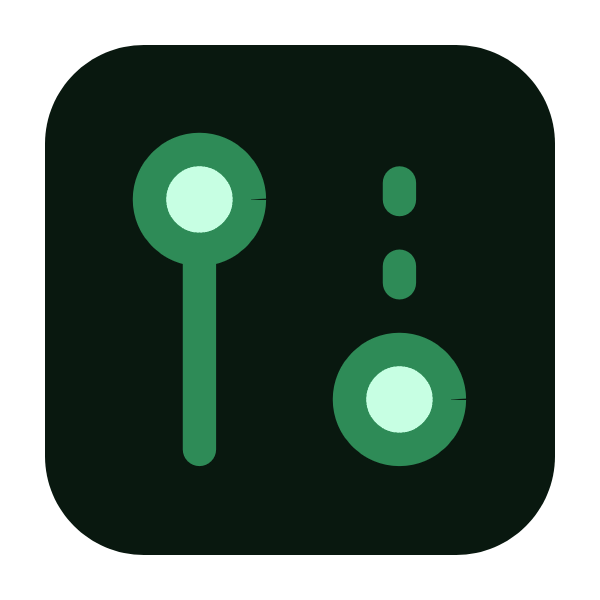
https://elm.0x3d.site/
Elm Online Directory

https://lua.0x3d.site/
Lua Online Directory
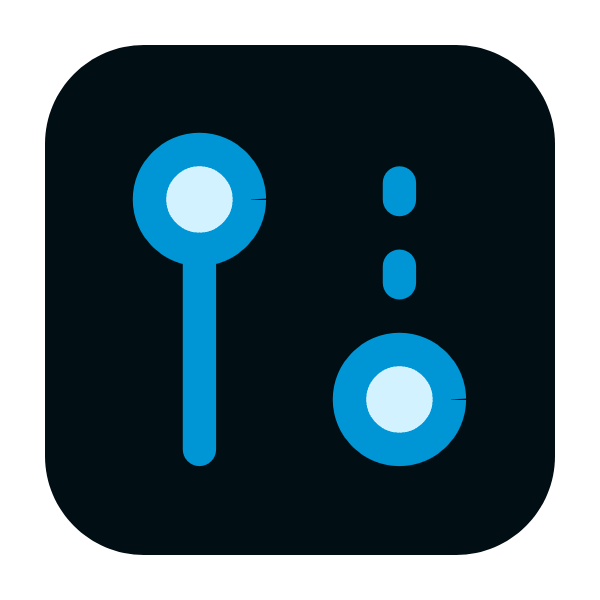
https://c-programming.0x3d.site/
C Programming Online Directory
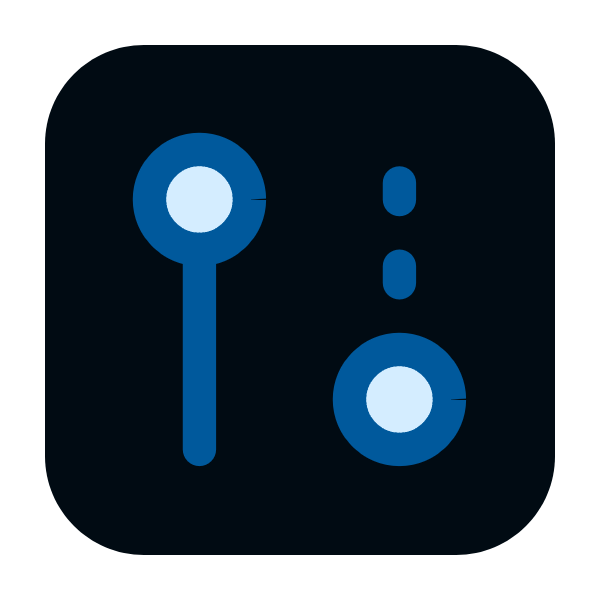
https://cpp-programming.0x3d.site/
C++ Programming Online Directory

https://r-programming.0x3d.site/
R Programming Online Directory

https://perl.0x3d.site/
Perl Online Directory
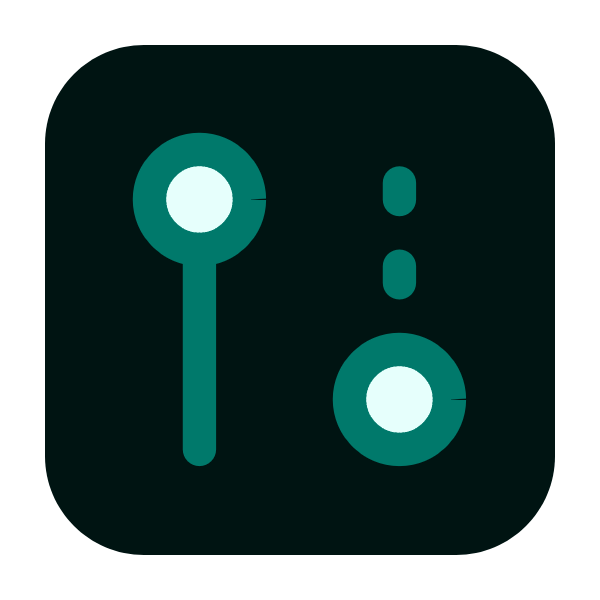
https://java.0x3d.site/
Java Online Directory

https://kotlin.0x3d.site/
Kotlin Online Directory

https://php.0x3d.site/
PHP Online Directory

https://react.0x3d.site/
React JS Online Directory
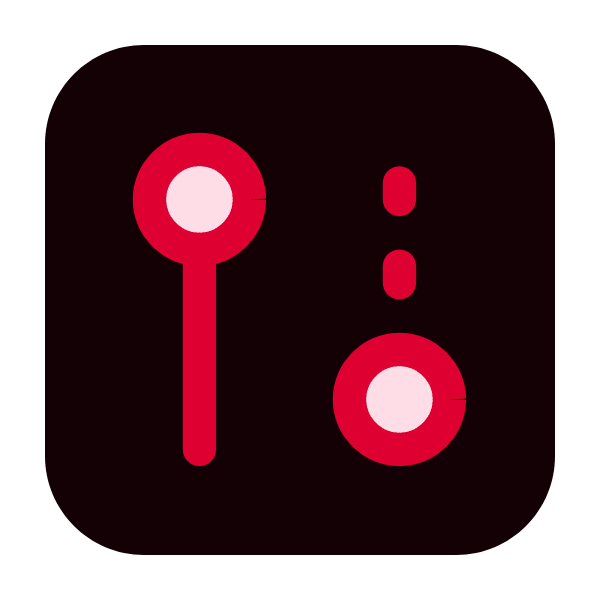
https://angular.0x3d.site/
Angular JS Online Directory